写一个生产者消费者的模拟程序设计的代码
时间: 2023-12-01 13:19:17 浏览: 28
好的,下面是一个简单的生产者消费者模拟程序的代码实现,使用了C++11的线程库和互斥量、条件变量等多线程同步机制:
```cpp
#include <iostream>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
using namespace std;
// 缓冲区大小
const int buffer_size = 10;
// 定义缓冲区
queue<int> buffer;
// 定义互斥锁和条件变量
mutex mtx;
condition_variable buffer_not_full;
condition_variable buffer_not_empty;
// 生产者线程函数
void producer(int id)
{
while (true) {
// 随机生成一个数据
int data = rand() % 100;
// 获取互斥锁
unique_lock<mutex> lock(mtx);
// 如果缓冲区满了,则等待缓冲区有空闲位置
while (buffer.size() == buffer_size) {
buffer_not_full.wait(lock);
}
// 添加数据到缓冲区
buffer.push(data);
cout << "Producer " << id << " produced data: " << data << endl;
// 通知等待缓冲区有数据的线程
buffer_not_empty.notify_all();
// 释放互斥锁
lock.unlock();
// 等待一段时间后再生产下一个数据
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
}
}
// 消费者线程函数
void consumer(int id)
{
while (true) {
// 获取互斥锁
unique_lock<mutex> lock(mtx);
// 如果缓冲区为空,则等待缓冲区有数据
while (buffer.empty()) {
buffer_not_empty.wait(lock);
}
// 从缓冲区取出数据
int data = buffer.front();
buffer.pop();
cout << "Consumer " << id << " consumed data: " << data << endl;
// 通知等待缓冲区有空闲位置的线程
buffer_not_full.notify_all();
// 释放互斥锁
lock.unlock();
// 等待一段时间后再消费下一个数据
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
}
}
int main()
{
// 创建多个生产者和消费者线程
vector<thread> producers;
vector<thread> consumers;
for (int i = 0; i < 2; i++) {
producers.emplace_back(producer, i);
consumers.emplace_back(consumer, i);
}
// 等待所有线程完成
for (auto& t : producers) {
t.join();
}
for (auto& t : consumers) {
t.join();
}
return 0;
}
```
上面的代码中,有两个生产者线程和两个消费者线程,并且生产者和消费者都是循环运行的。当缓冲区满了时,生产者会等待缓冲区有空闲位置的条件变量,当缓冲区为空时,消费者会等待缓冲区有数据的条件变量。每个线程在执行完任务后都会等待一段时间,模拟生产和消费的时间间隔。
相关推荐
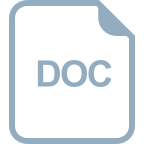
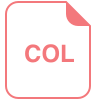













