vue 实现 大数据量 虚拟列表
时间: 2023-08-29 12:13:00 浏览: 107
虚拟列表是一种优化长列表性能的技术,它只渲染页面中可见的部分,而不是全部渲染。在 Vue 中,可以通过以下几个步骤来实现大数据量虚拟列表:
1. 确定列表项高度
在实现虚拟列表之前,需要先确定列表项的高度。这是因为虚拟列表需要根据列表项高度来计算可见区域的数量和位置。如果列表项高度不确定,可能会导致虚拟列表无法正确计算可见区域的位置。
2. 计算可见区域
虚拟列表需要计算出可见区域的位置和数量。可以通过以下公式来计算:
```js
const visibleCount = Math.ceil(containerHeight / itemHeight)
const startIndex = Math.floor(scrollTop / itemHeight)
```
其中,`visibleCount` 表示可见区域的数量,`containerHeight` 表示列表容器的高度,`itemHeight` 表示列表项的高度,`startIndex` 表示可见区域的起始索引,`scrollTop` 表示列表容器的滚动距离。
3. 动态渲染列表项
根据可见区域的位置和数量,可以动态渲染列表项。可以使用 `v-for` 指令来遍历列表数据,并使用 `v-show` 指令来控制列表项是否显示。例如:
```html
<div class="list-container" ref="listContainer" @scroll="handleScroll">
<div class="list" :style="{ height: listHeight + 'px' }">
<div v-for="(item, index) in visibleData" :key="item.id" :style="{ top: (startIndex + index) * itemHeight + 'px' }" v-show="index < visibleCount">
{{ item.text }}
</div>
</div>
</div>
```
其中,`listContainer` 表示列表容器的引用,`listHeight` 表示列表的总高度,`visibleData` 表示可见的列表数据,`startIndex` 表示可见区域的起始索引,`itemHeight` 表示列表项的高度,`visibleCount` 表示可见区域的数量。
4. 监听滚动事件
为了实现列表的滚动效果,需要监听列表容器的滚动事件,并根据滚动距离来计算可见区域的位置和数量。可以使用 `ref` 属性来获取列表容器的引用,并使用 `@scroll` 指令来监听滚动事件。例如:
```js
methods: {
handleScroll() {
const scrollTop = this.$refs.listContainer.scrollTop
const visibleCount = Math.ceil(this.$refs.listContainer.offsetHeight / this.itemHeight)
const startIndex = Math.floor(scrollTop / this.itemHeight)
this.visibleData = this.data.slice(startIndex, startIndex + visibleCount)
}
}
```
其中,`scrollTop` 表示列表容器的滚动距离,`visibleCount` 表示可见区域的数量,`startIndex` 表示可见区域的起始索引,`visibleData` 表示可见的列表数据,`data` 表示完整的列表数据。
通过以上步骤,就可以实现大数据量虚拟列表了。需要注意的是,虚拟列表只渲染可见区域的部分,因此在列表项中使用计算属性等复杂操作可能会影响性能。如果需要对列表项进行复杂操作,可以考虑使用 `watch` 监听列表数据的变化,然后重新计算可见区域并更新列表。
阅读全文
相关推荐
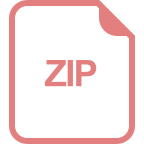
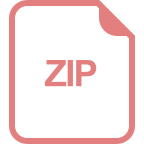
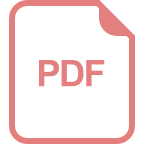
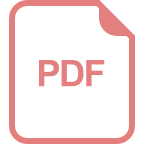
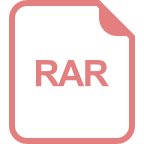
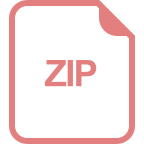
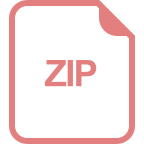
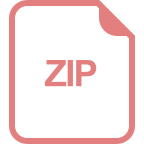
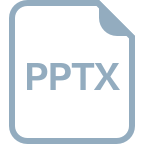
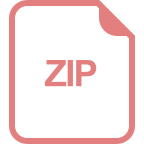
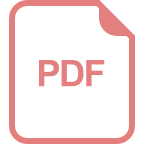
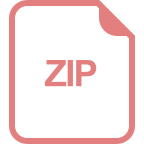
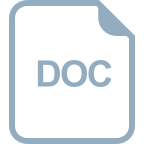
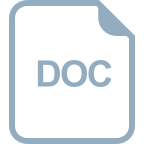
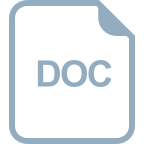
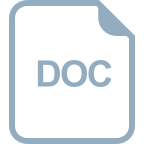