排课程序c++
时间: 2023-11-22 19:53:26 浏览: 49
排课程序是一个比较复杂的程序,需要考虑到多个因素,如教师的空闲时间、教室的可用情况、学生的选课情况等等。下面是一个简单的排课程序的示例代码,供参考:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义教室结构体
struct Classroom {
int id; // 教室编号
int capacity; // 教室容量
};
// 定义课程结构体
struct Course {
int id; // 课程编号
int teacher_id; // 授课教师编号
int classroom_id; // 授课教室编号
int start_time; // 开始时间
int end_time; // 结束时间
};
// 定义教师结构体
struct Teacher {
int id; // 教师编号
vector<int> available_times; // 教师空闲时间
};
// 比较函数,按照课程开始时间从小到大排序
bool cmp(Course a, Course b) {
return a.start_time < b.start_time;
}
int main() {
// 初始化教室
vector<Classroom> classrooms = {
{1, 50},
{2, 60},
{3, 70},
{4, 80}
};
// 初始化教师
vector<Teacher> teachers = {
{1, {1, 2, 5}},
{2, {1, 4, 6}},
{3, {2, 3, 4}},
{4, {3, 5, 6}}
};
// 初始化课程
vector<Course> courses = {
{1, 1, 1, 1, 2},
{2, 2, 2, 2, 3},
{3, 3, 3, 3, 4},
{4, 4, 4, 4, 5}
};
// 按照开始时间排序
sort(courses.begin(), courses.end(), cmp);
// 排课
for (auto course : courses) {
bool found = false;
for (auto teacher : teachers) {
if (teacher.id == course.teacher_id) {
// 找到对应的教师
for (auto time : teacher.available_times) {
// 在教师空闲时间内查找教室
for (auto classroom : classrooms) {
if (classroom.id == course.classroom_id && classroom.capacity >= course.capacity && time >= course.start_time && time + 1 <= course.end_time) {
// 找到对应的教室
cout << "课程 " << course.id << " 安排在教室 " << classroom.id << ",时间 " << time << "~" << time + 1 << ",由教师 " << teacher.id << " 授课。" << endl;
found = true;
break;
}
}
if (found) {
break;
}
}
if (found) {
break;
}
}
}
if (!found) {
cout << "无法安排课程 " << course.id << "。" << endl;
}
}
return 0;
}
```
以上示例代码仅供参考,实际的排课程序需要根据具体需求进行设计和实现。
阅读全文
相关推荐
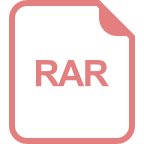
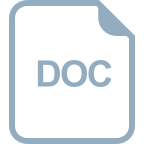















