用c语言帮我完成一个笔记管理系统,基本功能要求,实现基础界面:参照现有的笔记管理工具:打开笔记、关闭笔记、保存笔记、使用说明等内容;支持笔记创建,允许用户自主创建笔记以及命名;支持笔记编辑,允许用户对笔记内容进行编辑,文本输入;支持笔记查询,允许用户通过搜索功能查找所需的笔记
时间: 2023-08-14 12:02:40 浏览: 52
好的,您需要一个基于命令行界面的笔记管理系统,使用C语言实现。下面是一个简单的实现,您可以根据自己的需求进行修改和扩展。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NOTE_SIZE 10000 // 笔记最大长度
#define MAX_NOTE_NAME_SIZE 100 // 笔记名称最大长度
// 笔记结构体
typedef struct Note {
char name[MAX_NOTE_NAME_SIZE]; // 笔记名称
char content[MAX_NOTE_SIZE]; // 笔记内容
struct Note *next; // 指向下一个笔记的指针
} Note;
// 全局变量,指向笔记链表的头节点
Note *note_list = NULL;
// 显示菜单
void show_menu() {
printf("Welcome to Note Management System!\n");
printf("1. Open note\n");
printf("2. Create note\n");
printf("3. Edit note\n");
printf("4. Search note\n");
printf("5. Save note\n");
printf("6. Close note\n");
printf("7. Help\n");
printf("8. Exit\n");
}
// 创建新笔记
Note *create_note() {
Note *new_note = (Note *)malloc(sizeof(Note));
new_note->next = NULL;
printf("Please enter the name of the note: ");
scanf("%s", new_note->name);
printf("Please enter the content of the note: ");
getchar(); // 消耗掉之前输入的回车符
fgets(new_note->content, MAX_NOTE_SIZE, stdin);
return new_note;
}
// 打开笔记
void open_note() {
char name[MAX_NOTE_NAME_SIZE];
printf("Please enter the name of the note you want to open: ");
scanf("%s", name);
Note *p = note_list;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
printf("%s\n", p->content);
return;
}
p = p->next;
}
printf("Note not found.\n");
}
// 编辑笔记
void edit_note() {
char name[MAX_NOTE_NAME_SIZE];
printf("Please enter the name of the note you want to edit: ");
scanf("%s", name);
Note *p = note_list;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
printf("Please enter the new content of the note: ");
getchar(); // 消耗掉之前输入的回车符
fgets(p->content, MAX_NOTE_SIZE, stdin);
printf("Note updated.\n");
return;
}
p = p->next;
}
printf("Note not found.\n");
}
// 查找笔记
void search_note() {
char keyword[MAX_NOTE_SIZE];
printf("Please enter the keyword to search: ");
scanf("%s", keyword);
Note *p = note_list;
int count = 0;
while (p != NULL) {
if (strstr(p->content, keyword) != NULL) {
printf("%s\n", p->name);
count++;
}
p = p->next;
}
if (count == 0) {
printf("No note found.\n");
}
}
// 保存笔记
void save_note() {
char name[MAX_NOTE_NAME_SIZE];
printf("Please enter the name of the note you want to save: ");
scanf("%s", name);
Note *p = note_list;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
FILE *fp = fopen(name, "w");
if (fp == NULL) {
printf("Failed to save note.\n");
return;
}
fputs(p->content, fp);
fclose(fp);
printf("Note saved.\n");
return;
}
p = p->next;
}
printf("Note not found.\n");
}
// 关闭笔记
void close_note() {
printf("Note closed.\n");
}
// 帮助信息
void show_help() {
printf("Note Management System\n");
printf("Usage:\n");
printf("1. Open note: display the content of a note\n");
printf("2. Create note: create a new note\n");
printf("3. Edit note: edit the content of an existing note\n");
printf("4. Search note: search for notes containing a keyword\n");
printf("5. Save note: save the content of a note to a file\n");
printf("6. Close note: close the current note\n");
printf("7. Help: display this help message\n");
printf("8. Exit: exit the program\n");
}
int main() {
int choice = -1;
while (choice != 8) {
show_menu();
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1: // 打开笔记
open_note();
break;
case 2: // 创建笔记
Note *new_note = create_note();
new_note->next = note_list;
note_list = new_note;
printf("Note created.\n");
break;
case 3: // 编辑笔记
edit_note();
break;
case 4: // 查找笔记
search_note();
break;
case 5: // 保存笔记
save_note();
break;
case 6: // 关闭笔记
close_note();
break;
case 7: // 帮助信息
show_help();
break;
case 8: // 退出程序
printf("Goodbye!\n");
break;
default:
printf("Invalid choice.\n");
}
}
// 释放笔记链表的内存
Note *p = note_list;
while (p != NULL) {
Note *temp = p;
p = p->next;
free(temp);
}
return 0;
}
```
这个笔记管理系统具有基本的功能,可以进行笔记的创建、编辑、保存、打开、关闭和查询。您可以根据自己的需求进行修改和扩展,比如添加更多的菜单选项,支持多种文件格式的导入和导出等等。
相关推荐
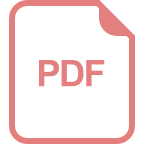














