设计异形迷宫,不同于传统的方形迷宫,异形迷宫 中每个迷宫单元是由相同的多边形组成,如图,是 一个六边形迷宫,每一个单元格与其它单元格最多 有六个通道可以连接。 1)自动生成并走出迷宫。 2)迷宫单元的边数由用户输入确定 c++
时间: 2024-05-04 17:22:41 浏览: 136
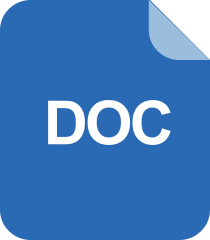
C++课程设计 迷宫
这个问题可以使用深度优先搜索(DFS)算法来生成迷宫。下面是一个简单的C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
struct Cell {
vector<int> neighbors; // 存储相邻单元格的索引
bool visited = false; // 标记单元格是否被访问过
};
vector<Cell> generateMaze(int numSides) {
vector<Cell> maze(numSides);
// 为每个单元格添加相邻单元格的索引
for (int i = 0; i < numSides; i++) {
Cell& cell = maze[i];
for (int j = 0; j < 6; j++) {
int neighborIndex = (i + j + 1) % numSides;
cell.neighbors.push_back(neighborIndex);
}
}
// 使用DFS算法遍历单元格并随机打通相邻的单元格
stack<int> stack;
stack.push(0);
maze[0].visited = true;
while (!stack.empty()) {
int currentIndex = stack.top();
stack.pop();
Cell& currentCell = maze[currentIndex];
for (int i = 0; i < currentCell.neighbors.size(); i++) {
int neighborIndex = currentCell.neighbors[i];
Cell& neighborCell = maze[neighborIndex];
if (!neighborCell.visited) {
currentCell.neighbors.erase(currentCell.neighbors.begin() + i);
neighborCell.neighbors.erase(find(neighborCell.neighbors.begin(), neighborCell.neighbors.end(), currentIndex));
stack.push(currentIndex);
stack.push(neighborIndex);
neighborCell.visited = true;
break;
}
}
}
return maze;
}
int main() {
int numSides;
cout << "请输入迷宫单元的边数:";
cin >> numSides;
vector<Cell> maze = generateMaze(numSides);
// 输出迷宫的连接情况
for (int i = 0; i < numSides; i++) {
cout << "单元格 " << i << " 连接的单元格:";
for (int j = 0; j < maze[i].neighbors.size(); j++) {
cout << maze[i].neighbors[j] << " ";
}
cout << endl;
}
return 0;
}
```
该示例程序中使用了一个 `Cell` 结构体来表示每个迷宫单元格,其中 `neighbors` 向量存储相邻单元格的索引。首先在 `generateMaze` 函数中初始化每个单元格的相邻单元格,并使用一个栈来实现DFS算法。在遍历过程中,如果当前单元格有未访问的相邻单元格,则随机选择一个相邻单元格并打通连接。最终输出每个单元格连接的单元格的索引。
请注意,此代码示例可能不是最优实现,但应该足以满足本题要求。
阅读全文
相关推荐
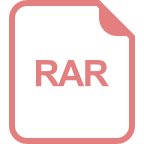

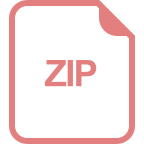
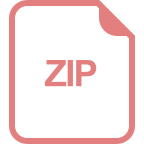
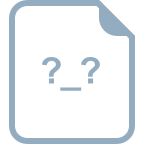
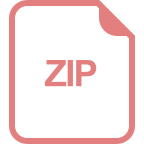
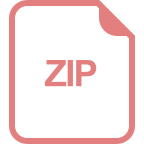
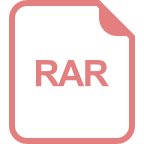
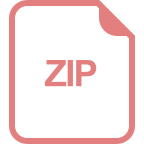
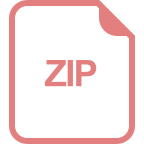