how does the programme following work:os.system("cls" if os.name == "nt" else "clear")
时间: 2023-11-23 10:07:28 浏览: 159
This program uses the os.system function to clear the console screen. The function takes a command as a parameter and executes it in the command prompt.
The command being executed is "cls" if the operating system is Windows (nt stands for "New Technology") and "clear" if it is Unix-based (such as Linux or macOS). This is because the command to clear the screen in the command prompt is different for these two types of systems.
So, this code checks the operating system being used and then executes the appropriate command to clear the console screen.
相关问题
ProgramModelEntities = _migrateRepository.GetAllWithNoTrackingAsync<ProgramModelEntity>().ToList(); Programmes = ProgramModelEntities.Select(program => { CProgramme p = new CProgramme() { Id = new Guid(program.Id), Name = program.Name, SchoolId = SQIConstants.SYS_School_ID, Description = program.Description, CreatedById = String.IsNullOrEmpty(program.CreatedBy) ? new Guid("e306ca07-a21b-40fd-935a-343cfe369001") : new Guid(program.CreatedBy), CreatedTime = program.CreatedOn == null ? new DateTime().Ticks : program.CreatedOn.Value.Ticks, ModifiedById = String.IsNullOrEmpty(program.ModifiedBy) ? new Guid("e306ca07-a21b-40fd-935a-343cfe369001") : new Guid(program.ModifiedBy), ModifiedTime = program.ModifiedOn == null ? new DateTime().Ticks : program.ModifiedOn.Value.Ticks, Duration = 1 }; if (!String.IsNullOrEmpty(program.ProgramAdmins)) { var admins = program.ProgramAdmins.Split(";"); ResourceAccesses.Add(admins.Select(admin => new CResourceAccess() { Id = Guid.NewGuid(), UserId = new Guid(admin), RoleType = RoleType.ProgrammeAdmin, ResourceId = new Guid(program.Id), ResourceLevel = ResourceLevel.Programme } ).ToList()); } ResourcesList.Add(new CResources { Id = new Guid(program.Id), ResourceLevel = ResourceLevel.Programme, SchoolId = SQIConstants.SYS_School_ID, ProgrammeId = new Guid(program.Id), }); return p; } ).ToList(); 优化下这段代码
以下是对这段代码的一些优化建议:
1. 使用异步方法来获取数据,可以提高性能。例如,可以使用 `ToListAsync()` 替代 `ToList()`。
2. 使用 LINQ 的 Select 方法来进行对象的转换,可以让代码更简洁易读。例如,可以使用如下的代码替代 `ProgramModelEntities.Select()`:
```
Programmes = await _migrateRepository.GetAllWithNoTrackingAsync<ProgramModelEntity>()
.Select(program => new CProgramme
{
Id = new Guid(program.Id),
Name = program.Name,
SchoolId = SQIConstants.SYS_School_ID,
Description = program.Description,
CreatedById = String.IsNullOrEmpty(program.CreatedBy)
? new Guid("e306ca07-a21b-40fd-935a-343cfe369001")
: new Guid(program.CreatedBy),
CreatedTime = program.CreatedOn?.Ticks ?? new DateTime().Ticks,
ModifiedById = String.IsNullOrEmpty(program.ModifiedBy)
? new Guid("e306ca07-a21b-40fd-935a-343cfe369001")
: new Guid(program.ModifiedBy),
ModifiedTime = program.ModifiedOn?.Ticks ?? new DateTime().Ticks,
Duration = 1
})
.ToListAsync();
```
3. 对于资源列表和资源访问列表的操作,可以使用 LINQ 的 SelectMany 方法和 Where 方法来替代 foreach 循环。例如,可以使用如下的代码替代 `if (!String.IsNullOrEmpty(program.ProgramAdmins))` 和 `ResourcesList.Add()`:
```
var programmeAdmins = program.ProgramAdmins?.Split(';');
if (programmeAdmins?.Any() == true)
{
ResourceAccesses.AddRange(programmeAdmins
.Select(admin => new CResourceAccess
{
Id = Guid.NewGuid(),
UserId = new Guid(admin),
RoleType = RoleType.ProgrammeAdmin,
ResourceId = new Guid(program.Id),
ResourceLevel = ResourceLevel.Programme
}));
}
ResourcesList.AddRange(programmeAdmins?.Select(admin => new CResources
{
Id = new Guid(program.Id),
ResourceLevel = ResourceLevel.Programme,
SchoolId = SQIConstants.SYS_School_ID,
ProgrammeId = new Guid(program.Id),
}) ?? Enumerable.Empty<CResources>());
```
综上所述,以下是经过优化后的代码:
```
Programmes = await _migrateRepository.GetAllWithNoTrackingAsync<ProgramModelEntity>()
.Select(program => new CProgramme
{
Id = new Guid(program.Id),
Name = program.Name,
SchoolId = SQIConstants.SYS_School_ID,
Description = program.Description,
CreatedById = String.IsNullOrEmpty(program.CreatedBy)
? new Guid("e306ca07-a21b-40fd-935a-343cfe369001")
: new Guid(program.CreatedBy),
CreatedTime = program.CreatedOn?.Ticks ?? new DateTime().Ticks,
ModifiedById = String.IsNullOrEmpty(program.ModifiedBy)
? new Guid("e306ca07-a21b-40fd-935a-343cfe369001")
: new Guid(program.ModifiedBy),
ModifiedTime = program.ModifiedOn?.Ticks ?? new DateTime().Ticks,
Duration = 1
})
.ToListAsync();
var programmeResources = Programmes.SelectMany(programme => new[]
{
new CResources
{
Id = programme.Id,
ResourceLevel = ResourceLevel.Programme,
SchoolId = SQIConstants.SYS_School_ID,
ProgrammeId = programme.Id
}
});
var programmeAdminResources = Programmes.SelectMany(programme =>
{
var programmeAdmins = programme.ProgramAdmins?.Split(';');
return programmeAdmins?.Select(admin => new CResourceAccess
{
Id = Guid.NewGuid(),
UserId = new Guid(admin),
RoleType = RoleType.ProgrammeAdmin,
ResourceId = programme.Id,
ResourceLevel = ResourceLevel.Programme
}) ?? Enumerable.Empty<CResourceAccess>();
});
ResourcesList.AddRange(programmeResources);
ResourceAccesses.AddRange(programmeAdminResources);
```
11.写出执行abc.sh文件的命令以及执行后的结果:文件名:abc.sh#!/bin/bash shell类型#a simple shell script example#a functionfunciton sayhello() 函数{echo "Enter Your name:"read name 读取来自键盘输入的变量echo "Hello $name"}echo "programme starts here..." 主过程sayhelloecho "programme ends."
执行abc.sh文件的命令是:`./abc.sh`
执行后的结果是:
```
programme starts here...
Enter Your name:
[在这里输入你的名字,比如"Tom"]
Hello Tom
programme ends.
```
阅读全文
相关推荐
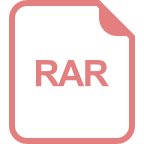
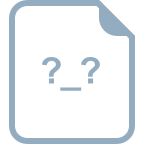
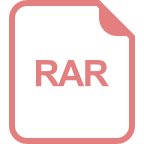
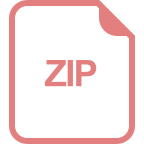









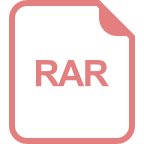
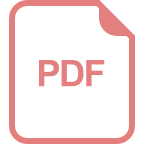
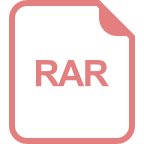