Use java to create a new class that reads the conversation.txt line by line and prints it to the console. In this class you should implement two different methods to read the file:1. Implement a method using a Scanner that reads the file2. Implement a method that uses a BufferedReader to read the file.Finally, alter the second method that reads the file line by line using a BufferedReader. After printing the first two lines, make your programme wait for the user to press enter before printing the next two lines. Continue this until all the lines are printed. Once the end of the file is reached, the programme should terminate so that when the user presses enter again, nothing bad happens.
时间: 2023-07-02 22:15:14 浏览: 90
Sure, here's the code to implement the above requirements:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class ReadConversation {
public static void main(String[] args) throws IOException {
// Method 1: Using Scanner to read file
File file = new File("conversation.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
scanner.close();
System.out.println("\n---------------------------------\n");
// Method 2: Using BufferedReader to read file
FileReader fileReader = new FileReader(file);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line;
int count = 0;
int linesToPrint = 2;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
count++;
if (count == linesToPrint) {
System.out.print("Press Enter to continue...");
Scanner inputScanner = new Scanner(System.in);
inputScanner.nextLine();
count = 0;
}
}
bufferedReader.close();
}
}
```
This code reads the file "conversation.txt" using two different methods - `Scanner` and `BufferedReader`. The first method simply reads the file and prints it line by line. The second method reads the file line by line using `BufferedReader` and waits for the user to press enter after printing every two lines.
Hope this helps!
相关推荐
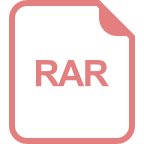
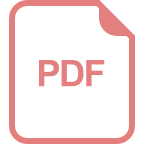
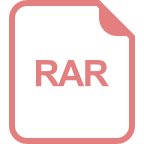














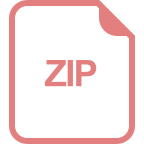