Write a C++ program that reads the strings from file SortedStrings. txt and report whether the strings in the files are stored. in ascending order. If the strings are not sorted in the file, display the first two strings that are out of order. Please use string class to do this task. SortedStrings.txt stores multiple lines of strings like: a1 a2 b1 b2 c1 c2
时间: 2024-02-07 12:01:51 浏览: 99
Here's a possible C++ program to solve the problem:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream infile("SortedStrings.txt");
string prev_str, curr_str;
bool sorted = true;
// read the first string from the file
if (getline(infile, prev_str)) {
// read the rest of the strings and check if they are in ascending order
while (getline(infile, curr_str)) {
if (curr_str < prev_str) {
sorted = false;
cout << "The strings in the file are not sorted in ascending order.\n";
cout << "The first two out-of-order strings are:\n";
cout << prev_str << endl << curr_str << endl;
break;
}
prev_str = curr_str;
}
}
if (sorted) {
cout << "The strings in the file are sorted in ascending order.\n";
}
infile.close();
return 0;
}
```
In this program, we first open the file "SortedStrings.txt" using an ifstream object. We then read the first string from the file using getline() function, and store it in the variable prev_str. We then start reading the rest of the strings from the file using another getline() function, and compare each string with the previous string. If we find a string that is smaller than the previous string, we set the sorted flag to false, and display the first two out-of-order strings. Otherwise, we continue reading the file until the end, and if all strings are sorted, we display a message indicating that the strings are sorted in ascending order.
Note that in this program, we have used the string class to store the strings read from the file, and the < operator to compare two strings. This is possible because the string class overloads the < operator to perform lexicographic comparison of two strings.
阅读全文
相关推荐
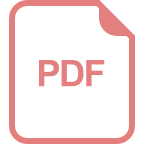















