Write the while loop that reads each line from the console input stream and prints the line out to the file. Each line should be prepended with the count of the line number. in java
时间: 2024-01-06 10:04:55 浏览: 100
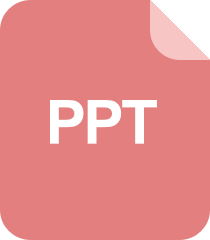
java stream
Here's an example of a while loop in Java that reads each line from the console input stream and prints the line out to a file, prepended with the count of the line number:
```java
import java.io.*;
public class LineNumberedFileWriter {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
FileWriter writer = new FileWriter("output.txt");
String line;
int lineNumber = 1;
while ((line = reader.readLine()) != null) {
writer.write(lineNumber + ": " + line + "\n");
lineNumber++;
}
reader.close();
writer.close();
}
}
```
In this example, we first create a `BufferedReader` object to read lines from the console input stream, and a `FileWriter` object to write the lines to a file called "output.txt". We also initialize a `lineNumber` variable to keep track of the line number.
Then, we enter a while loop that reads each line from the input stream using the `readLine()` method of the `BufferedReader`. If the line is not null (i.e., there are still more lines to read), we write the line to the file using the `write()` method of the `FileWriter`, prepended with the current line number and a colon. We also increment the `lineNumber` variable.
Finally, we close both the input stream and the file writer using the `close()` method to free up system resources.
阅读全文
相关推荐
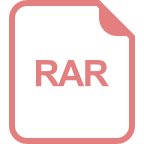
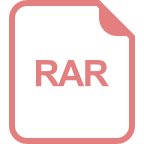










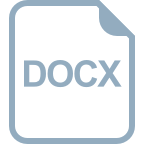
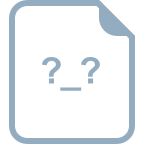
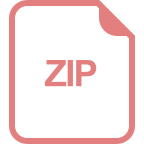