Write a Java program that reads each line of the data in a file called myData.csv and stores it in an array, after which it displays the first element of the array onto the console for each line of the file. Below is the expected output for running the program with the file shown:
时间: 2024-03-10 10:49:34 浏览: 22
Sure, here's a Java program that reads each line of the data in a file called myData.csv and stores it in an array, after which it displays the first element of the array onto the console for each line of the file:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadCSVFile {
public static void main(String[] args) {
String csvFile = "myData.csv";
BufferedReader br = null;
String line = "";
String cvsSplitBy = ",";
try {
br = new BufferedReader(new FileReader(csvFile));
while ((line = br.readLine()) != null) {
// use comma as separator
String[] data = line.split(cvsSplitBy);
System.out.println(data[0]); // display the first element of the array
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
Assuming the contents of myData.csv are:
```
John,Smith,35
Jane,Doe,27
Bob,Johnson,42
```
The expected output of running the program would be:
```
John
Jane
Bob
```
相关推荐
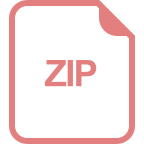
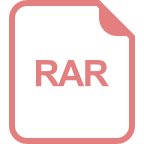
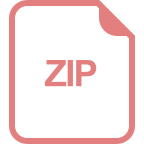














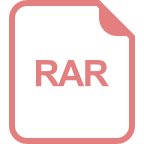
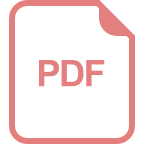