In this exercise you will create a program to read text from standard input and write it to a file with each line prefixed with a line-number count. This file will be specified by a command-line argument. Directions Create a program called TestLineNumbering that accepts one command-line argument, the name of a file. Your code should verify that the command-line argument was specified. Declare a variable that holds a File object that is initialized from the file named in the first command-line argument. Within a try-catch block, create a buffered, input stream reader based on the System.in stream (standard input). Also, create a print file writer based on the File variable. Write the while loop that reads each line from the console input stream and prints the line out to the file. Each line should be prepended with the count of the line number. Make sure to close the I/O streams.
时间: 2024-01-06 19:03:50 浏览: 34
Here is a possible solution in Java:
```
import java.io.*;
public class TestLineNumbering {
public static void main(String[] args) {
if (args.length != 1) {
System.err.println("Usage: java TestLineNumbering <output-file>");
System.exit(1);
}
String fileName = args[0];
try {
File outputFile = new File(fileName);
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
PrintWriter writer = new PrintWriter(outputFile);
String line;
int lineNumber = 0;
while ((line = reader.readLine()) != null) {
writer.printf("%d: %s%n", ++lineNumber, line);
}
writer.close();
reader.close();
} catch (IOException e) {
System.err.println("Error: " + e.getMessage());
System.exit(2);
}
}
}
```
The program checks that exactly one command-line argument is provided, which is the name of the output file. It then creates a `BufferedReader` to read from standard input, and a `PrintWriter` to write to the output file. The program reads each line from standard input, increments a line number counter, and writes the line with the line number prefix to the output file. Finally, the I/O streams are closed, and any exceptions are caught and reported.
相关推荐
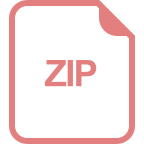
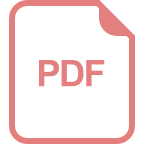
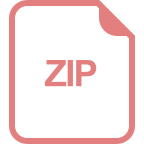














