解释这段代码:#include<iostream> #include<string> using namespace std; int trie[1000010][26]; // using an array to implement the trie int num[1000010] = { 0 }; // the number of words prefixed with a certain string int pos = 1; // for the storage position of a string void Insert(char str[]) { // insert str to the trie int c = 0; for (int i = 0; str[i]; i++) { int n = str[i] - 'a'; if (trie[c][n] == 0) // if there is no corresponding character trie[c][n] = pos++; c = trie[c][n]; num[c]++; } } int Find(char str[]) { // return the number of words prefixed with str int c = 0; for (int i = 0; str[i]; i++) { int n = str[i] - 'a'; if (trie[c][n] == 0) return 0; c = trie[c][n]; } return num[c]; } int main() { char str[11]; while (getline(cin,str)) { if (!strlen(str)) break; // input an empty line Insert(str); } while (gets(str)) cout << Find(str) << endl; return 0; }
时间: 2024-04-25 17:26:28 浏览: 71
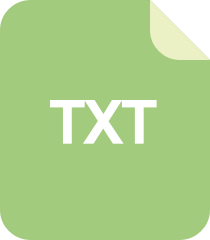
#include_iostream.h_

这段代码是实现了一个 Trie 树(字典树),用于字符串的存储和查找。Trie 树是一种树形结构,用于存储一组字符串,每个节点表示一个字符串的前缀,从根节点到某个节点的路径表示一个字符串。代码中使用一个二维数组 trie 来实现 Trie 树,其中 trie[i][j] 表示第 i 个节点的第 j 个子节点的编号。使用 num 数组记录每个节点代表的字符串在 Trie 树中出现的次数。
具体实现中,Insert 函数用于向 Trie 树中插入字符串。对于每个字符,从根节点开始向下遍历,若存在对应的子节点,则将遍历指针移向该子节点,否则新建一个节点。每经过一个节点,就将该节点代表的字符串出现次数加1。
Find 函数用于查找以给定字符串为前缀的字符串的数量。同样是从根节点开始向下遍历,若遇到某个字符没有对应的子节点,则说明 Trie 树中不存在以该字符串为前缀的字符串,返回0;否则返回最后一个字符所在节点的出现次数。
主函数中,先读入一些字符串,将其插入 Trie 树中。然后从标准输入中读取一些字符串,查找以其为前缀的字符串的数量,并输出结果。
阅读全文
相关推荐
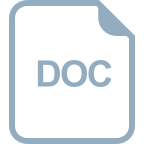
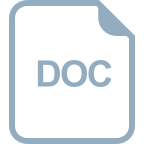


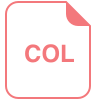











