用c++解决下述问题:描述 Given some words, can you figure out if there are words prefixed with a string? You should notice that the word itself is a prefix of it. All the letters will be lowercase ones. 输入 The first part of the input is a word list. The length of each word will not exceed 10. Then a blank line follows which means the end of the word list. The second part includes queries, and each query is a string. The sum of their lengths will not exceed 10^6. There will be only one test case in each input file. 输出 For each test case, if there's a word (or words) prefixed with the string, print "YES", otherwise print "NO".
时间: 2024-02-14 12:11:38 浏览: 153
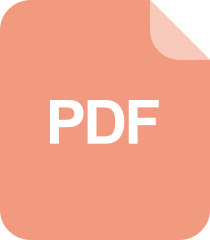
C++问题解决办法.pdf
以下是用 C++ 解决上述问题的代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
struct TrieNode {
vector<TrieNode*> children;
bool isEndOfWord;
TrieNode() : children(26, NULL), isEndOfWord(false) {}
};
void insert(TrieNode* root, const string& word) {
TrieNode* curr = root;
for (char c : word) {
if (curr->children[c - 'a'] == NULL) {
curr->children[c - 'a'] = new TrieNode();
}
curr = curr->children[c - 'a'];
}
curr->isEndOfWord = true;
}
bool search(TrieNode* root, const string& word) {
TrieNode* curr = root;
for (char c : word) {
if (curr->children[c - 'a'] == NULL) {
return false;
}
curr = curr->children[c - 'a'];
}
return curr->isEndOfWord;
}
bool startsWith(TrieNode* root, const string& prefix) {
TrieNode* curr = root;
for (char c : prefix) {
if (curr->children[c - 'a'] == NULL) {
return false;
}
curr = curr->children[c - 'a'];
}
return true;
}
int main() {
TrieNode* root = new TrieNode();
string word;
while (getline(cin, word) && !word.empty()) {
insert(root, word);
}
string query;
while (cin >> query) {
if (startsWith(root, query)) {
cout << "YES" << endl;
} else {
cout << "NO" << endl;
}
}
return 0;
}
```
这里使用了 Trie 树来实现字符串的前缀匹配。首先读入所有单词,然后对于每个查询字符串,判断是否是某个单词的前缀,如果是则输出 "YES",否则输出 "NO"。
阅读全文
相关推荐
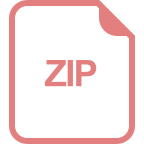

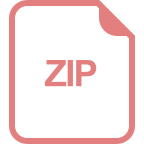
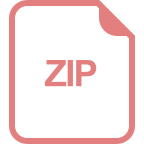
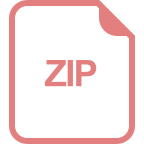
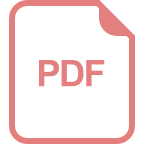
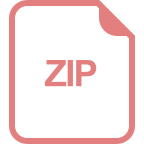
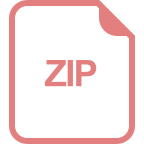
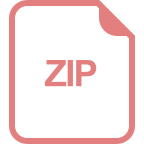
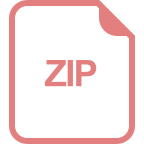
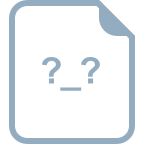
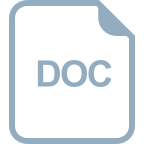


