用c++和trie解决下述问题:描述 Given some words, can you figure out if there are words prefixed with a string? You should notice that the word itself is a prefix of it. All the letters will be lowercase ones. 输入 The first part of the input is a word list. The length of each word will not exceed 10. Then a blank line follows which means the end of the word list. The second part includes queries, and each query is a string. The sum of their lengths will not exceed 10^6. There will be only one test case in each input file. 输出 For each test case, if there's a word (or words) prefixed with the string, print "YES", otherwise print "NO".
时间: 2024-02-14 11:11:42 浏览: 78
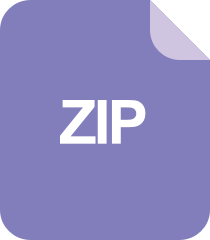
trie:Trie 是一个用 C++11 编写的轻量级和简单的自动补全数据结构
以下是使用 C++ 和 Trie 数据结构解决该问题的代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class TrieNode {
public:
TrieNode() : is_word(false), children(26, nullptr) {}
void insert(const string& word) {
TrieNode* node = this;
for (char c : word) {
int index = c - 'a';
if (!node->children[index]) {
node->children[index] = new TrieNode();
}
node = node->children[index];
}
node->is_word = true;
}
bool search(const string& word) const {
const TrieNode* node = this;
for (char c : word) {
int index = c - 'a';
if (!node->children[index]) {
return false;
}
node = node->children[index];
}
return node->is_word;
}
private:
bool is_word;
vector<TrieNode*> children;
};
int main() {
TrieNode root;
// 读取单词列表并将其插入到 Trie 中
string word;
while (getline(cin, word)) {
if (word.empty()) {
break;
}
root.insert(word);
}
// 对于每个查询字符串,搜索 Trie 中以其为前缀的单词
string query;
while (cin >> query) {
cout << (root.search(query) ? "YES" : "NO") << endl;
}
return 0;
}
```
该程序首先定义了一个 TrieNode 类,用于表示 Trie 中的一个节点。每个节点包含一个布尔值 is_word,表示从根节点到该节点的路径上是否形成一个单词,以及一个长度为 26 的子节点数组 children,用于存储该节点的子节点。类中定义了两个方法:insert() 用于将一个单词插入到 Trie 中,search() 用于搜索 Trie 中是否存在以给定字符串为前缀的单词。
程序主函数首先创建一个 Trie 根节点,并读取一系列单词,并将它们插入到 Trie 中。然后,对于每个查询字符串,搜索 Trie 中以该字符串为前缀的单词。如果找到了这样的单词,则输出“YES”,否则输出“NO”。
阅读全文
相关推荐
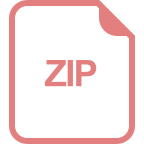
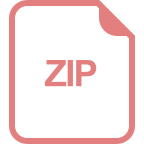

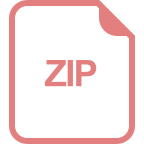
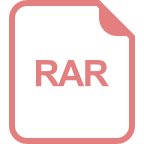
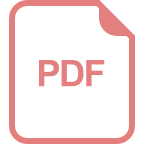
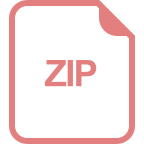
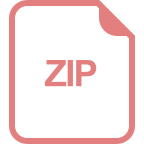
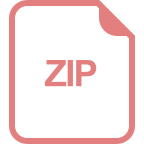
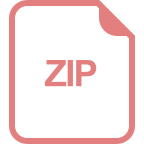
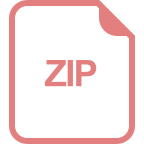
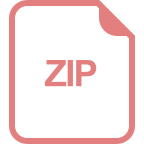
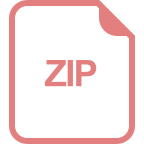
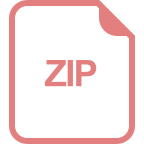
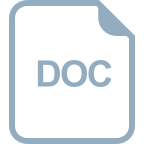