用C语言实现用递归法将一个整数n转换成字符串
时间: 2024-10-26 12:16:46 浏览: 32
在C语言中,你可以使用递归函数将整数转换为字符串。这里是一个简单的示例,它定义了一个名为`itoa()`的递归函数,该函数接受一个整数`num`和一个指向数组`str`的指针,然后逐步构建字符串表示形式:
```c
#include <stdio.h>
#include <string.h>
// Recursive function to convert an integer to a string
char* itoa(int num, char* str, int radix) {
if (num == 0) { // Base case: number is zero, append '0'
str[0] = '0';
str[1] = '\0'; // null terminator
return str;
} else if (num < 0) { // Negative numbers are prefixed with '-'
str[0] = '-';
num = -num;
}
// Calculate the next digit and its position in the string
int digit = num % radix;
str[0] = (digit < 10) ? (digit + '0') : ('A' + digit - 10); // digits 10-35 map to A-Z
str[1] = '\0';
// Move to the next position and call recursively for the quotient
return itoa(num / radix, str + 2, radix);
}
int main() {
int n = 12345;
char result[20]; // Assuming the largest possible integer is less than 2^16
itoa(n, result, 10);
printf("The string representation of %d is: %s\n", n, result);
return 0;
}
```
阅读全文
相关推荐
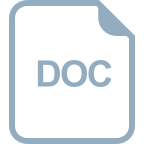
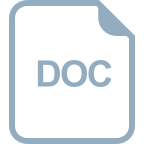
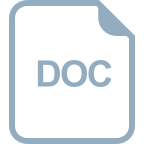















