写一个c语言程序,用递归法将一个整数n转换成字符串。n可以是任意位数的正整数
时间: 2024-05-14 12:15:50 浏览: 110
好的,这里是这个问题的C语言程序,使用了递归方法将一个整数转换为字符串:
```c
#include <stdio.h>
#include <string.h>
void intToStr(int num, char str[], int index) {
if (num == 0) {
str[index] = '\0'; // 加上字符串结束符号
return;
}
intToStr(num / 10, str, index - 1);
str[index] = (char)((num % 10) + '0'); // 将数字转换成对应的字符
}
int main() {
int num = 12345;
char str[20];
intToStr(num, str, strlen(str) - 1);
printf("The integer %d is converted to string %s\n", num, str);
return 0;
}
```
在主函数中,我们定义了一个整数num = 12345,并且定义了一个字符串数组str,长度为20。我们使用一个名为“intToStr”的递归函数将整数num转换为字符串,并存储在str数组中。在递归过程中,我们首先将整数除以10,然后递归调用intToStr将商转换为字符串,并将余数转换为字符添加到字符串的末尾。
最后,我们在主函数中打印出转换后的字符串。
相关问题
c语言用递归法将一个整数n转换成字符串。例如输入483,应输出字符串“483”。n的位数不确定,可以是任意数位的正整数”
以下是用递归法将一个整数n转换成字符串的C语言代码实现:
```c
#include <stdio.h>
void int_to_str(int n, char str[])
{
if (n == 0) {
str[0] = '0';
str[1] = '\0';
return;
}
if (n < 0) {
str[0] = '-';
int_to_str(-n, str+1);
return;
}
int i = 0, temp = n;
while (temp > 0) {
temp /= 10;
i++;
}
str[i] = '\0';
while (n > 0) {
str[--i] = n % 10 + '0';
n /= 10;
}
}
int main()
{
int n = -483;
char str[20];
int_to_str(n, str);
printf("The string is %s\n", str);
return 0;
}
```
首先处理特殊情况,如果n为0,则直接将字符串设为“0”;如果n为负数,则在字符串首位加上符号“-”,并递归处理其绝对值。然后计算n的位数,并将字符串末尾设为NULL,最后按照从高位到低位的顺序将每一位数字转换成字符存储到字符串中。
C语言用递归法将一个整数n转换成字符串
可以使用递归的方式将一个整数n转换成字符串,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
void intToStr(int n, char *str)
{
if (n == 0) {
*str = '0';
*(str + 1) = '\0';
return;
}
if (n < 0) {
*str = '-';
n = -n;
str++;
}
int len = 0;
int temp = n;
while (temp > 0) {
len++;
temp /= 10;
}
for (int i = 0; i < len; i++) {
*(str + i) = n % 10 + '0';
n /= 10;
}
int i = 0;
if (*str == '-') {
i = 1;
}
int j = len - 1;
while (i < j) {
char tmp = *(str + i);
*(str + i) = *(str + j);
*(str + j) = tmp;
i++;
j--;
}
*(str + len) = '\0';
}
int main()
{
int n = -12345;
char *str = (char *)malloc(20 * sizeof(char));
intToStr(n, str);
printf("n: %d, str: %s\n", n, str);
free(str);
return 0;
}
```
在实现中,首先判断n的正负性,并将负数的符号写入字符串中,然后计算n的位数,将每一位的数字转换为字符并存储到字符串中,最后将字符串反转即可。注意要在字符串的结尾处添加'\0'。
阅读全文
相关推荐
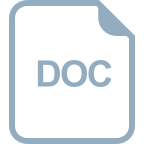
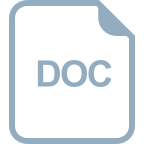
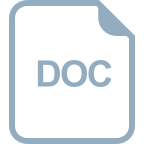


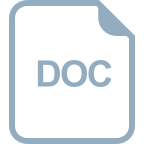
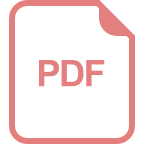
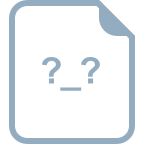
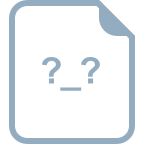
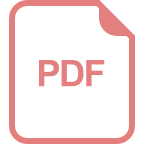
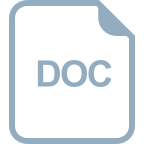
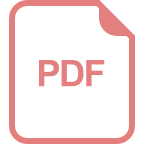
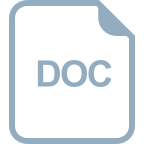
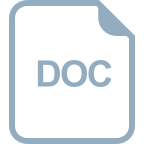
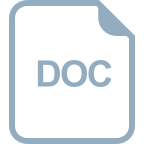


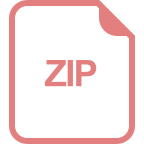