Write a program that reads the file created in (1) above. Display the names and numbers on the screen in a suitable format.,display the names and numbers with the names in alphabetical order. usec++
时间: 2023-06-25 13:04:05 浏览: 114
Here's a sample C++ program that reads a file containing names and numbers, displays them on the screen, and sorts them alphabetically by name:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
struct Contact {
string name;
string number;
};
bool compareContacts(const Contact& c1, const Contact& c2) {
return c1.name < c2.name;
}
int main() {
ifstream infile("contacts.txt");
vector<Contact> contacts;
if (infile) {
string line;
while (getline(infile, line)) {
Contact contact;
size_t pos = line.find(',');
if (pos != string::npos) {
contact.name = line.substr(0, pos);
contact.number = line.substr(pos + 1);
contacts.push_back(contact);
}
}
infile.close();
// Display the names and numbers
cout << "Contacts:\n";
for (const auto& contact : contacts) {
cout << contact.name << ": " << contact.number << "\n";
}
// Sort the contacts by name
sort(contacts.begin(), contacts.end(), compareContacts);
// Display the sorted names and numbers
cout << "\nSorted Contacts:\n";
for (const auto& contact : contacts) {
cout << contact.name << ": " << contact.number << "\n";
}
} else {
cout << "Unable to open file\n";
}
return 0;
}
```
Assuming the file is named "contacts.txt" and has the following contents:
```
John Doe,555-1234
Jane Smith,555-5678
Bob Johnson,555-9012
```
The output of the program would be:
```
Contacts:
John Doe: 555-1234
Jane Smith: 555-5678
Bob Johnson: 555-9012
Sorted Contacts:
Bob Johnson: 555-9012
Jane Smith: 555-5678
John Doe: 555-1234
```
阅读全文
相关推荐













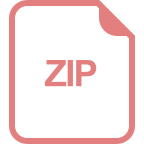
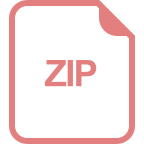
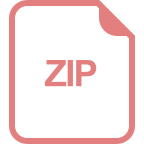