Write a program that reads the integers between 1 and 100 and counts the occurrences of each. Assume the input ends with 0. 用Java实现
时间: 2024-09-16 12:03:44 浏览: 80
当然可以。下面是一个简单的Java程序,它会读取用户输入的1到100之间的整数,并统计每个数字出现的次数。当遇到0时,程序会停止计数并打印结果。
```java
import java.util.HashMap;
import java.util.Map;
public class IntegerCount {
public static void main(String[] args) {
Map<Integer, Integer> countMap = new HashMap<>();
Scanner scanner = new Scanner(System.in);
int num;
while (true) {
System.out.print("Enter an integer between 1 and 100 (or type 0 to stop): ");
num = scanner.nextInt();
if (num == 0) {
break; // 如果输入的是0,则跳出循环
}
countMap.put(num, countMap.getOrDefault(num, 0) + 1); // 更新计数
}
System.out.println("\nCounts:");
for (Map.Entry<Integer, Integer> entry : countMap.entrySet()) {
System.out.println("Number " + entry.getKey() + " appears " + entry.getValue() + " times.");
}
scanner.close();
}
}
```
这个程序首先创建了一个HashMap来存储数字及其对应的计数。然后通过Scanner读取用户的输入,如果输入的数字是0,则结束循环。不是0的话,就更新HashMap中的计数。最后,遍历HashMap并打印出每个数字及其出现的次数。
阅读全文
相关推荐
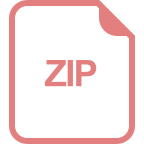
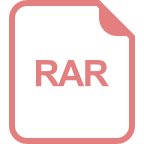
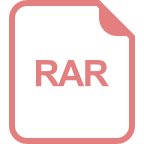














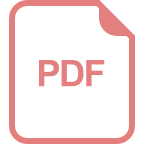
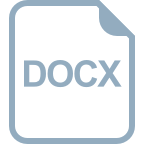