Write a program which reads two integers x and y, and prints them in ascending order. 输入 The input consists of multiple datasets. Each dataset consists of two integers x and y separated by a single space. The input ends with two 0 (when both x and y are zero). Your program should not process for these terminal symbols. 输出 For each dataset, print x and y in ascending order in a line. Put a single space between x and y.
时间: 2023-06-20 19:10:21 浏览: 257
这是一个简单的排序题目,我们只需要读入两个整数,然后将它们排序后输出即可。
下面是一个 Python 的实现:
```python
while True:
x, y = map(int, input().split())
if x == 0 and y == 0:
break
if x > y:
x, y = y, x
print(x, y)
```
首先,我们使用 `while` 循环不断读入数据,直到读入两个 0 停止循环。在每次循环中,我们使用 `input()` 函数读入一行输入,并使用 `split()` 方法将其拆分成两个字符串,然后使用 `map()` 函数和 `int()` 函数将字符串转换为整数,并将其赋值给变量 `x` 和 `y`。
接着,我们使用一个 `if` 语句判断 `x` 是否大于 `y`,如果是,则交换 `x` 和 `y` 的值。最后,我们使用 `print()` 函数输出 `x` 和 `y` 的值,中间用一个空格隔开。
注意,本题要求输出时需要有一个空格隔开 `x` 和 `y`,而且每组数据输出一行,因此我们需要在输出时使用 `print()` 函数,并将 `x` 和 `y` 作为参数传递给它。
相关问题
用c++写Write a program which reads two integers x and y, and prints them in ascending order. 输入 The input consists of multiple datasets. Each dataset consists of two integers x and y separated by a single space. The input ends with two 0 (when both x and y are zero). Your program should not process for these terminal symbols. 输出 For each dataset, print x and y in ascending order in a line. Put a single space between x and y.
以下是用 C++ 编写的程序:
```c++
#include <iostream>
using namespace std;
int main() {
int x, y;
while (cin >> x >> y) {
if (x == 0 && y == 0) break; // 终止条件
if (x > y) swap(x, y); // 交换 x 和 y
cout << x << " " << y << endl;
}
return 0;
}
```
程序的逻辑很简单,就是读入两个整数 x 和 y,然后比较它们的大小,将它们按升序输出。当输入的 x 和 y 均为 0 时,程序结束。
用c++写In the online judge system, a judge file may include multiple datasets to check whether the submitted program outputs a correct answer for each test case. This task is to practice solving a problem with multiple datasets. Write a program which reads an integer x and print it as is. Note that multiple datasets are given for this problem. 输入 The input consists of multiple datasets. Each dataset consists of an integer x in a line. The input ends with an integer 0. You program should not process (print) for this terminal symbol. 输出 For each dataset, print x in the following format: Case i: x where i is the case number which starts with 1. Put a single space between "Case" and i. Also, put a single space between ':' and x.
以下是用 C++ 编写的解题代码:
```c++
#include <iostream>
using namespace std;
int main() {
int x, i = 1;
while (cin >> x && x != 0) {
cout << "Case " << i << ": " << x << endl;
i++;
}
return 0;
}
```
这个程序使用了一个 while 循环来不断读入输入数据,直到读入的数据为 0 为止。在循环中,我们输出当前的 case 编号和输入的整数值。需要注意的是,每个 case 编号后面要输出一个冒号和一个空格。
在输出时,我们使用了 `endl` 来输出一个换行符。这样做可以确保每组数据的输出都占用一行,并且在输出结束后换行,方便阅读。
阅读全文
相关推荐
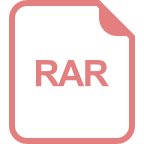
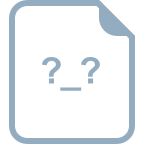












