Write a program that reads an expression in a line as input and prints out the result. Only integers and operators below are allowed in the expression: + - * / % ( ) 输入格式: A line of expression. 输出格式: The result.
时间: 2024-02-07 10:02:34 浏览: 104
好的,我可以为您提供一个基于 Python 的解决方案。请注意,此程序仅适用于输入格式符合要求的表达式。
```python
# 定义算术运算函数
def arithmetic(op, a, b):
if op == '+':
return a + b
elif op == '-':
return a - b
elif op == '*':
return a * b
elif op == '/':
return a / b
elif op == '%':
return a % b
# 定义运算符优先级
precedence = {'+': 1, '-': 1, '*': 2, '/': 2, '%': 2}
# 定义中缀表达式转后缀表达式函数
def infix_to_postfix(expr):
stack = []
postfix = []
for token in expr:
if token.isdigit():
postfix.append(token)
elif token in precedence:
while stack and stack[-1] != '(' and precedence[token] <= precedence[stack[-1]]:
postfix.append(stack.pop())
stack.append(token)
elif token == '(':
stack.append(token)
elif token == ')':
while stack and stack[-1] != '(':
postfix.append(stack.pop())
stack.pop()
while stack:
postfix.append(stack.pop())
return postfix
# 定义计算后缀表达式函数
def evaluate_postfix(expr):
stack = []
for token in expr:
if token.isdigit():
stack.append(int(token))
elif token in precedence:
b = stack.pop()
a = stack.pop()
stack.append(arithmetic(token, a, b))
return stack.pop()
# 读取表达式并输出结果
expr = input().split()
postfix = infix_to_postfix(expr)
result = evaluate_postfix(postfix)
print(result)
```
您可以将上述代码复制粘贴到 Python 编辑器中,然后运行程序。程序将提示您输入表达式,并输出计算结果。例如,如果您输入 "2 + 3 * 4", 程序将输出 14。
阅读全文
相关推荐
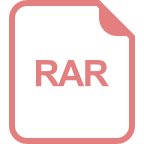













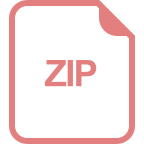
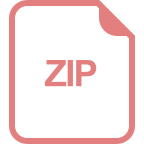
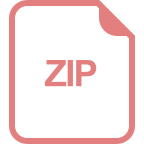