Write a program that counts the occurrences of words in a text and displays the words and their occurrences in alphabetical order of words. The program uses a TreeMap to store an entry consisting a wo
时间: 2024-03-25 14:40:29 浏览: 70
rd and its count. Here's an example program in Java:
```java
import java.util.Scanner;
import java.util.TreeMap;
public class WordCounter {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
TreeMap<String, Integer> wordCountMap = new TreeMap<>();
System.out.println("Enter text (press Ctrl+D to end):");
while (scanner.hasNext()) {
String word = scanner.next().toLowerCase().replaceAll("[^a-z]+", "");
if (!word.isEmpty()) {
int count = wordCountMap.getOrDefault(word, 0);
wordCountMap.put(word, count + 1);
}
}
System.out.println("Word count:");
for (String word : wordCountMap.keySet()) {
System.out.println(word + " " + wordCountMap.get(word));
}
}
}
```
The program reads text from standard input using a `Scanner` and stores the word counts in a `TreeMap<String, Integer>`. Each word is converted to lowercase and stripped of non-alphabetic characters using a regular expression. The `getOrDefault` method is used to retrieve the current count for a word, and `put` is used to update the count. Finally, the program outputs the word counts in alphabetical order of words using a `for` loop over the key set of the `TreeMap`.
相关推荐
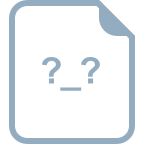










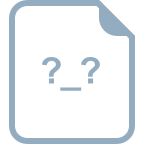
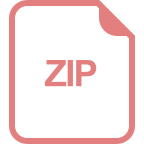