A program counts the number of words in a string read from the user,and then exits. __ pattern is most suitable for implementing this specification
时间: 2024-03-05 07:51:57 浏览: 22
The simplest pattern to implement the given specification is the linear pattern, also known as the straight-line pattern. The program reads the input string, counts the number of words, and then exits. There are no branches, loops, or other control structures involved. Here is an example implementation in Python using the linear pattern:
```python
sentence = input("Enter a sentence: ")
words = len(sentence.split())
print("The sentence has", words, "words.")
```
In this implementation, the input sentence is split into words using the split() method and then the number of words is counted using the len() function. Finally, the number of words is printed to the console and the program exits.
相关问题
Write a program that asks a string to the user, and counts the number of time each character occurs in the string,output is a set
Sure, I can help you with that! Here's a Python program that does what you're asking for:
```
string = input("Please enter a string: ")
count = {}
for char in string:
if char in count:
count[char] += 1
else:
count[char] = 1
print("Character count:")
for char, num in count.items():
print(char, num)
```
This program prompts the user to enter a string, then creates a dictionary called `count` to keep track of how many times each character appears in the string. It loops through each character in the string, and if the character is already in the `count` dictionary, it increments the count by 1. If the character is not yet in the dictionary, it adds it with a count of 1. Finally, it prints out the character count for each character in the string.
Write a program that takes a string input from the user and counts the number of unique characters in the string. Note:The program should only count each unique character once, even if it appears multiple times i n the input string. The program should als
o ignore spaces and special characters.
Sure, here's a Python program that does what you asked:
```
def count_unique_chars(string):
unique_chars = set()
for char in string:
if char.isalnum():
unique_chars.add(char.lower())
return len(unique_chars)
user_input = input("Enter a string: ")
print("Number of unique characters:", count_unique_chars(user_input))
```
Explanation:
- The `count_unique_chars` function takes a string as input and returns the number of unique characters in it.
- We create an empty set called `unique_chars` to keep track of unique characters as we iterate through the string.
- For each character in the string, we check if it is alphanumeric (i.e., a letter or a number), and convert it to lowercase using the `lower()` method. If it is, we add it to the `unique_chars` set.
- Finally, we return the length of the `unique_chars` set, which gives us the number of unique characters in the string.
- In the main program, we prompt the user to enter a string, and then call the `count_unique_chars` function on it. The result is printed to the console.
I hope this helps! Let me know if you have any further questions.
相关推荐
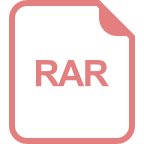
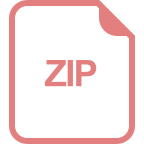
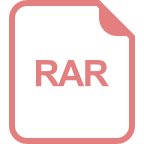












