How can I create a program or function that counts the number of repetitions in a given input string or sequence?
时间: 2024-10-25 07:12:52 浏览: 17
创建一个计算给定输入字符串或序列中字符重复次数的程序或函数,你可以遵循这些步骤:
1. **选择编程语言**:首先确定你要使用的编程语言,例如 Python、JavaScript 或 C++ 等。
2. **初始化数据结构**:
- Python: 使用字典 (dictionary) 存储每个字符及其计数。
- JavaScript: 使用 Map 或 Object 存储。
- C++: 可能需要哈希表 (unordered_map) 或数组 + 记录索引。
3. **遍历输入**:
- 对于字符串,遍历每一个字符。
- 对于序列,遍历序列中的每一个元素。
4. **更新计数**:
- 如果字符或元素已经在数据结构中,则增加其计数。
- 如果不存在,添加新项并设置计数为1。
5. **返回结果**:
- 最终的数据结构应该包含每个字符或元素及其重复次数。
这里是几个示例代码片段(假设你使用的是Python):
```python
def count_repetitions(input_string):
char_count = {}
for char in input_string:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
return char_count
# 或者更简洁地使用 collections.Counter
from collections import Counter
def count_repetitions_counter(input_sequence):
return Counter(input_sequence)
```
阅读全文
相关推荐
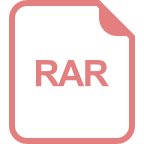
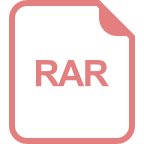
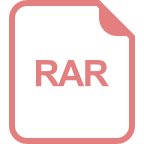















