Write a program in C to count the digits of a given number using recursion. Input: Input a non-negtive integer n. Output: Print the number of digits in the number n. Input Sample: 50 Output Sample: The number of digits in 50 is: 2
时间: 2024-01-03 12:04:54 浏览: 40
Sure, here's a program in C that counts the digits of a given number using recursion:
```c
#include <stdio.h>
// Function to count the number of digits in a number using recursion
int countDigits(int n) {
if (n == 0) {
return 0;
} else {
return 1 + countDigits(n / 10);
}
}
int main() {
int n;
// Read input from user
printf("Enter a non-negative integer: ");
scanf("%d", &n);
// Count the number of digits using recursion
int numDigits = countDigits(n);
// Print the result
printf("The number of digits in %d is: %d\n", n, numDigits);
return 0;
}
```
The `countDigits()` function takes an integer `n` as input and recursively counts the number of digits in `n` by dividing it by 10 until `n` becomes 0. The base case is when `n` is 0, in which case the function returns 0. Otherwise, the function returns 1 plus the result of calling itself with `n/10`.
In the `main()` function, we read the input from the user, call the `countDigits()` function to count the number of digits, and print the result.
相关推荐
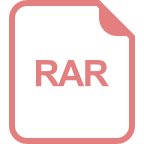
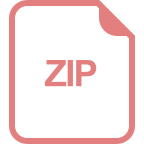
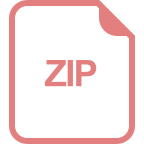













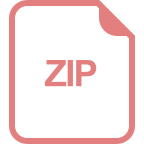