Write a program to find all numbers between 'a' and 'b' (both included) such that each digit of the number is an even number, and print the results. Input There are two non-negative integers in one line: a and b Output Directly print the list
时间: 2024-01-12 12:02:35 浏览: 24
Sure, here's a Python program that will solve the problem:
```python
a, b = map(int, input().split())
# Define a function to check if a number has all even digits
def all_even_digits(n):
while n > 0:
if (n % 10) % 2 != 0:
return False
n //= 10
return True
# Loop through all numbers between a and b, check if they have all even digits, and print them if they do
for i in range(a, b+1):
if all_even_digits(i):
print(i)
```
To use this program, simply input the values of 'a' and 'b' on the first line, separated by a space. The program will then output a list of all numbers between 'a' and 'b' (inclusive) that have all even digits.
相关问题
Write a python function proddigits() that inputs a positive integer number and returns the product of digits of that number.
Here's a Python function `proddigits()` that takes a positive integer as input and returns the product of its digits:
```python
def proddigits(number):
product = 1
while number > 0:
digit = number % 10
product *= digit
number //= 10
return product
```
This function uses a while loop to iterate through each digit of the input number. It calculates the product of each digit by using the modulus operator (%) to get the last digit and then dividing the number by 10 to shift the digits to the right. The loop continues until the number becomes 0.
Here's an example of how to use this function:
```python
number = 12345
result = proddigits(number)
print("Product of digits of", number, "is", result)
```
This would output:
```
Product of digits of 12345 is 120
```
which is the product of the digits 1 x 2 x 3 x 4 x 5.
Write a program to print all digits of a given number by using a recursive function.
Here's the Python code to print all digits of a given number using a recursive function:
```
def print_digits(n):
if n < 10:
print(n)
else:
print_digits(n // 10)
print(n % 10)
# Example usage:
print_digits(12345) # Output: 1 2 3 4 5
```
In this code, the `print_digits` function takes an integer `n` as input. If `n` is less than 10, we simply print it. Otherwise, we first call the `print_digits` function recursively with `n // 10`, which gives us all the digits except for the last one. Then, we print the last digit by computing `n % 10`. This way, we print the digits in reverse order (from right to left).
相关推荐
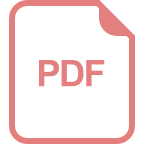
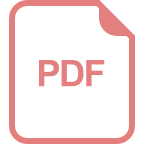












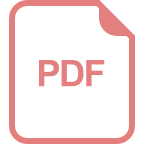