Write an object-oriented program that takes two digits and prints them in the output. a. The program must consist of two classes A and B, in which B is a subclass of A b. Each class should have a constructor and destructor. c. The first digit is read by the first class and the second digit is read by the second class d. The constructor of the derived class must call the constructor of the base class.
时间: 2024-02-04 10:04:19 浏览: 28
Sure, here's a possible implementation of the program in Python:
```python
class A:
def __init__(self):
self.num1 = None
def read_num1(self):
self.num1 = int(input("Enter the first digit: "))
def __del__(self):
print("Class A has been deleted.")
class B(A):
def __init__(self):
super().__init__()
self.num2 = None
def read_num2(self):
self.num2 = int(input("Enter the second digit: "))
def print_nums(self):
print("The first digit is:", self.num1)
print("The second digit is:", self.num2)
def __del__(self):
print("Class B has been deleted.")
# Testing the program
b = B()
b.read_num1()
b.read_num2()
b.print_nums()
```
In this program, class A and class B represent the reading of the first digit and the second digit, respectively. Class B is a subclass of class A, so it can use the methods defined in class A. Class B also adds a method to read the second digit and print both digits.
In the testing part of the program, we create an instance of class B and use it to read and print both digits. When the program finishes running, the destructor of class A and class B will be called to clean up memory and release resources.
I hope this implementation helps!
相关推荐
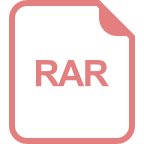
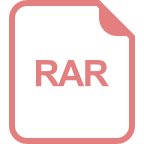













