write a Java program called SumOfDigits which reads an integer between 0 and 1000 and adds all the digits in the integer.
时间: 2024-02-18 22:04:15 浏览: 24
Sure, here's the Java program to solve your problem:
```
import java.util.Scanner;
public class SumOfDigits {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer between 0 and 1000: ");
int num = input.nextInt();
int sum = 0;
while (num > 0) {
sum += num % 10;
num /= 10;
}
System.out.println("The sum of the digits is " + sum);
}
}
```
This program prompts the user to enter an integer between 0 and 1000 and then uses a while loop to add up all the digits in the integer. The program then prints out the sum of the digits.
相关问题
Write a program that reads an expression in a line as input and prints out the result. Only integers and operators below are allowed in the expression:
Sure, I can help you with that! Here is a Python code snippet that can evaluate simple arithmetic expressions containing only integers and the operators +, -, *, and /:
```python
expression = input("Enter an arithmetic expression: ")
result = eval(expression)
print("Result:", result)
```
You can copy and paste this code into a Python IDE or a Jupyter notebook and execute it. When prompted, enter an arithmetic expression like "2+3*4" and press Enter. The program will then evaluate the expression and print out the result, which in this case should be 14.
Note that the `eval()` function is used to evaluate the expression entered by the user as a Python expression. This means that the user can potentially enter any valid Python expression, so you should be careful when using this function in a production environment.
Write a Java program that reads each line of the data in a file called myData.csv and stores it in an array, after which it displays the first element of the array onto the console for each line of the file. Below is the expected output for running the program with the file shown:
Sure, here's a Java program that reads each line of the data in a file called myData.csv and stores it in an array, after which it displays the first element of the array onto the console for each line of the file:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadCSVFile {
public static void main(String[] args) {
String csvFile = "myData.csv";
BufferedReader br = null;
String line = "";
String cvsSplitBy = ",";
try {
br = new BufferedReader(new FileReader(csvFile));
while ((line = br.readLine()) != null) {
// use comma as separator
String[] data = line.split(cvsSplitBy);
System.out.println(data[0]); // display the first element of the array
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
Assuming the contents of myData.csv are:
```
John,Smith,35
Jane,Doe,27
Bob,Johnson,42
```
The expected output of running the program would be:
```
John
Jane
Bob
```
相关推荐
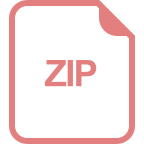
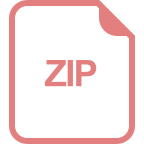
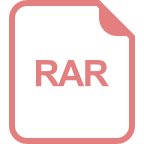












