Notice that the number 123456789 is a 9-digit number consisting exactly the numbers from 1 to 9, with no duplication. Double it we will obtain 246913578, which happens to be another 9-digit number consisting exactly the numbers from 1 to 9, only in a different permutation. Check to see the result if we double it again! Now you are suppose to check if there are more numbers with this property. That is, double a given number with k digits, you are to tell if the resulting number consists of only a permutation of the digits in the original number. Input Specification: Each input contains one test case. Each case contains one positive integer with no more than 20 digits. Output Specification: For each test case, first print in a line "Yes" if doubling the input number gives a number that consists of only a permutation of the digits in the original number, or "No" if not. Then in the next line, print the doubled number. Sample Input: 1234567899 Sample Output: Yes 2469135798
时间: 2023-12-29 08:03:54 浏览: 47
To solve this problem, we can first check if the number of digits in the given number is even or odd. If it is odd, then we can immediately output "No" and the doubled number. Otherwise, we can count the number of occurrences of each digit in the original number and the doubled number. If they match, then the doubled number consists of only a permutation of the digits in the original number; otherwise, it does not.
Here's the Python code:
```python
num = input().strip()
double_num = str(int(num) * 2)
if len(num) % 2 == 1:
print("No")
print(double_num)
else:
num_count = [0] * 10
double_count = [0] * 10
for digit in num:
num_count[int(digit)] += 1
for digit in double_num:
double_count[int(digit)] += 1
if num_count == double_count:
print("Yes")
else:
print("No")
print(double_num)
```
Note that we convert the doubled number to a string so that we can count the occurrences of each digit using a list of counts. We also use the `strip()` method to remove any leading or trailing whitespace in the input.
相关推荐
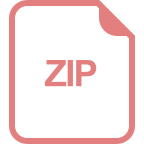
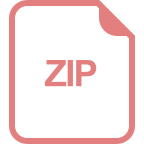













