Write a Python program that counts the number of vowels in a given string using a for loop.
时间: 2024-10-26 11:02:59 浏览: 36
当然可以。下面是一个简单的Python程序,它会使用for循环来计算给定字符串中元音字母的数量:
```python
def count_vowels(s):
vowels = "aeiouAEIOU" # 定义包含所有元音字母的字符串
vowel_count = 0 # 初始化元音计数器
for char in s: # 遍历输入字符串中的每个字符
if char in vowels: # 如果字符是元音
vowel_count += 1 # 增加计数
return vowel_count # 返回最终的元音数量
# 测试函数
input_string = "Hello, World!"
vowel_count = count_vowels(input_string)
print(f"The number of vowels in '{input_string}' is {vowel_count}.")
```
这个程序首先定义了一个包含所有元音的字符串,然后通过遍历输入字符串并检查每个字符是否属于元音,累加计数器。最后,它打印出指定字符串中的元音总数。
相关问题
write a python function that counts the number of positive factors of a positive integer
Sure, here's a Python function that counts the number of positive factors of a positive integer:
```python
def count_factors(n):
count = 0
for i in range(1, n+1):
if n % i == 0:
count += 1
return count
```
You can use this function by calling it with a positive integer as an argument, like this:
```python
count_factors(12) # Returns 6, since 12 has 6 positive factors: 1, 2, 3, 4, 6, and 12
count_factors(7) # Returns 2, since 7 has 2 positive factors: 1 and 7
```
c语言Write a function that counts the number of occurrences of a given character in a string.
在 C 语言中,你可以编写一个函数来计算给定字符在一个字符串中出现的次数。下面是一个简单的示例函数 `count_char` 的实现:
```c
#include <stdio.h>
#include <string.h>
int count_char(char *str, char target) {
int count = 0;
while (*str != '\0') { // 遍历字符串直到遇到结束符
if (*str == target) { // 如果当前字符等于目标字符
count++; // 增加计数器
}
str++; // 移动指针到下一个字符
}
return count; // 返回字符计数
}
// 示例用法
int main() {
char str[] = "Hello, World!";
char target = 'l';
printf("The character '%c' appears %d times in the string.\n", target, count_char(str, target));
return 0;
}
```
这个函数接受一个字符串 `str` 和一个字符 `target`,通过遍历字符串并检查每个字符是否与目标字符相等,最后返回该字符在字符串中出现的次数。
阅读全文
相关推荐
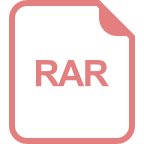
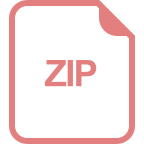
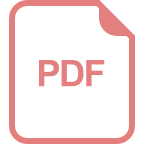











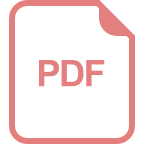
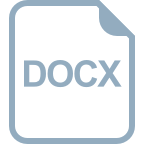
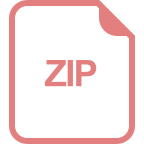