Write a c++ program that takes a string input from the user and counts the number of unique characters in the string. Note:The program should only count each unique character once, even if it appears multiple times i n the input string. The program should also ignore any whitespace characters or special characters t hat are not letters or numbers.
时间: 2024-02-14 17:09:07 浏览: 93
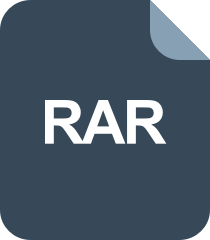
fibo.rar_The Program
Sure, here's a C++ program that takes a string input from the user and counts the number of unique characters in the string:
```c++
#include <iostream>
#include <string>
#include <unordered_set>
int main() {
std::string input;
std::getline(std::cin, input);
std::unordered_set<char> unique_chars;
for (char c : input) {
if (std::isalnum(c)) {
unique_chars.insert(c);
}
}
std::cout << "Number of unique characters: " << unique_chars.size() << std::endl;
return 0;
}
```
Here's how this program works:
- We start by reading a string input from the user using `std::getline`.
- We then create an `std::unordered_set` to store the unique characters in the input string. `std::unordered_set` is a container that stores unique elements, and allows for fast lookup and insertion.
- We iterate through each character in the input string using a range-based for loop. For each character, we check if it is alphanumeric (i.e. a letter or number) using `std::isalnum`. If it is, we insert it into the `unique_chars` set using `insert`.
- Finally, we print out the number of unique characters in the string using `unique_chars.size()`.
Note that this program ignores any whitespace characters or special characters that are not letters or numbers, as specified in the problem statement.
阅读全文
相关推荐
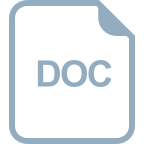
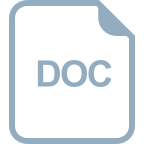















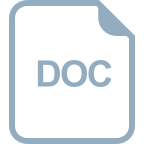