use C program Enter a string, count and output the number of capital consonants in the string (capital consonants: capital letters except A, E, I, O, U). 【Input】 Enter a character string. 【Output】 Output the number of uppercase consonants.
时间: 2024-02-29 19:51:05 浏览: 98
Sure, here's a C program that asks the user to input a string and counts the number of capital consonants (capital letters except A, E, I, O, U) in the string:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, count=0;
printf("Enter a string: ");
fgets(str, 100, stdin);
for(i=0; str[i]!='\0'; i++) {
if(isupper(str[i]) && str[i]!='A' && str[i]!='E' && str[i]!='I' && str[i]!='O' && str[i]!='U') {
count++;
}
}
printf("Number of capital consonants: %d\n", count);
return 0;
}
```
The program first declares a character array `str` of size 100 to store the user input. It then prompts the user to enter a string using the `printf()` function and reads the input using the `fgets()` function.
The program then loops through each character in the string using a `for` loop. For each character, it checks if it is an uppercase letter using the `isupper()` function, and also checks if it is not one of the vowels A, E, I, O, or U. If both conditions are true, then the program increments the `count` variable by 1.
Finally, the program prints the number of capital consonants using the `printf()` function.
I hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
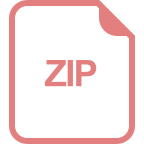
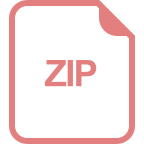
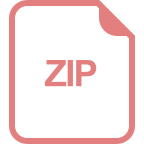
















