C++ 使用find_first_of 和find_last_of的代码例子
时间: 2023-11-20 18:04:55 浏览: 80
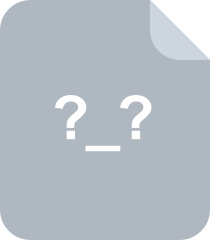
实例的C++例子
下面是使用 `find_first_of` 和 `find_last_of` 的代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "hello world";
char vowels[] = "aeiou";
char consonants[] = "bcdfghjklmnpqrstvwxyz";
// 使用 find_first_of 查找第一个元音字母
char* vowel_pos = strpbrk(str, vowels);
if (vowel_pos != NULL) {
printf("第一个元音字母是 %c\n", *vowel_pos);
}
// 使用 find_last_of 查找最后一个辅音字母
char* consonant_pos = strpbrk(str, consonants);
while (consonant_pos != NULL) {
char* next_consonant_pos = strpbrk(consonant_pos + 1, consonants);
if (next_consonant_pos == NULL) {
printf("最后一个辅音字母是 %c\n", *consonant_pos);
break;
}
consonant_pos = next_consonant_pos;
}
return 0;
}
```
在上面的代码中,我们使用 `strpbrk` 函数来查找字符串中的元音字母和辅音字母。`strpbrk` 函数在 C 标准库中定义,它的原型如下:
```c
char* strpbrk(const char* str1, const char* str2);
```
`str1` 是要查找的字符串,`str2` 是要在 `str1` 中查找的字符集合。`strpbrk` 函数返回指向 `str1` 中第一个出现在 `str2` 中的字符的指针,如果没有找到,则返回 `NULL`。
阅读全文
相关推荐
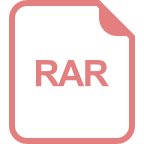
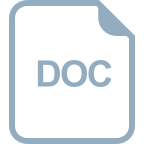



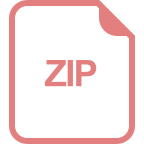
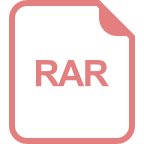
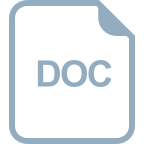
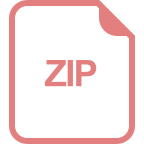
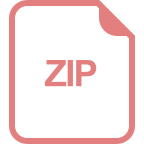
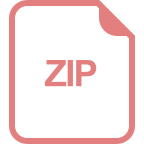
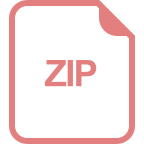
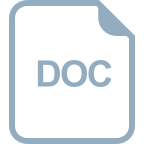


