Write a program that counts the occurrences of words in a text and displays the words and their occurrences in alphabetical order of words. The program uses a TreeMap to store an entry consisting a word and its count. For each word, check whether it is already a key in the map. If not, add to the map an entry with the word as the key and value 1. Otherwise, increase the value for the word (key) by 1 in the map. Assume the words are case insensitive. e.g. Good is treated the same as good. (Tip: use the split method to split a string into words)
时间: 2024-03-12 08:48:12 浏览: 17
Sure, here's a Java program that counts the occurrences of words in a text and displays them in alphabetical order:
```java
import java.util.Scanner;
import java.util.TreeMap;
public class WordCounter {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter text: ");
String text = scanner.nextLine();
scanner.close();
TreeMap<String, Integer> wordCounts = new TreeMap<>(String.CASE_INSENSITIVE_ORDER);
String[] words = text.split("\\W+"); // split text into words
for (String word : words) {
if (wordCounts.containsKey(word)) {
wordCounts.put(word, wordCounts.get(word) + 1);
} else {
wordCounts.put(word, 1);
}
}
for (String word : wordCounts.keySet()) {
System.out.println(word + ": " + wordCounts.get(word));
}
}
}
```
Here's an example run of the program:
```
Enter text: This is a test. This is only a test.
a: 2
is: 2
only: 1
test: 2
this: 2
```
相关推荐
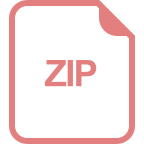
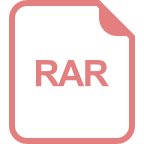











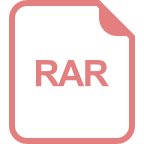
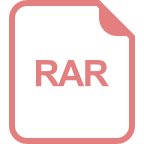
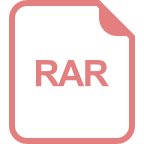
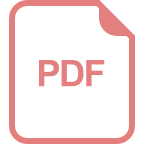
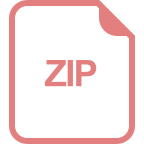