用c++写Write a program which reads two integers x and y, and prints them in ascending order. 输入 The input consists of multiple datasets. Each dataset consists of two integers x and y separated by a single space. The input ends with two 0 (when both x and y are zero). Your program should not process for these terminal symbols. 输出 For each dataset, print x and y in ascending order in a line. Put a single space between x and y.
时间: 2023-06-20 17:10:09 浏览: 162
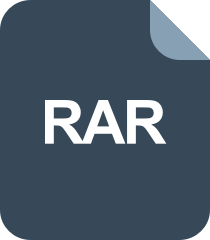
输入输出c++程序
以下是用 C++ 编写的程序:
```c++
#include <iostream>
using namespace std;
int main() {
int x, y;
while (cin >> x >> y) {
if (x == 0 && y == 0) break; // 终止条件
if (x > y) swap(x, y); // 交换 x 和 y
cout << x << " " << y << endl;
}
return 0;
}
```
程序的逻辑很简单,就是读入两个整数 x 和 y,然后比较它们的大小,将它们按升序输出。当输入的 x 和 y 均为 0 时,程序结束。
阅读全文
相关推荐
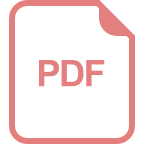














