Create a new class that reads the conversation.txt line by line and prints it to the console. In this class you should implement two different methods to read the file: 1. Implement a method using a Scanner that reads the file. (Successfully printing file content to Console: 1P) 2. Implement a method that uses a BufferedReader to read the file. (Successfully printing file content to Console: 1P) Finally, alter the second method that reads the file line by line using a BufferedReader. After printing the first two lines, make your programme wait for the user to press enter before printing the next two lines. Continue this until all the lines are printed. Once the end of the file is reached, the programme should terminate so that when the user presses enter again, nothing bad happens
时间: 2024-02-02 17:05:03 浏览: 71
Thank you for your question! Here's some code that should do what you've asked for:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class FileReadingExample {
public static void main(String[] args) throws IOException {
// Read the file using a Scanner
System.out.println("Reading file using Scanner:");
File file = new File("conversation.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
scanner.close();
// Read the file using a BufferedReader
System.out.println("\nReading file using BufferedReader:");
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
// Read the file using a BufferedReader with user input pauses
System.out.println("\nReading file using BufferedReader with user input pauses:");
reader = new BufferedReader(new FileReader(file));
int lineCount = 0;
while ((line = reader.readLine()) != null) {
System.out.println(line);
lineCount++;
if (lineCount % 2 == 0) {
// Wait for user to press enter before printing next two lines
Scanner inputScanner = new Scanner(System.in);
inputScanner.nextLine();
inputScanner.close();
}
}
reader.close();
}
}
```
This code first reads the file using a Scanner and prints its contents to the console. It then reads the file again using a BufferedReader and prints its contents to the console. Finally, it reads the file using a BufferedReader and waits for the user to press enter before printing the next two lines.
阅读全文
相关推荐
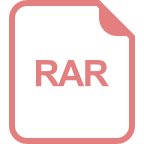
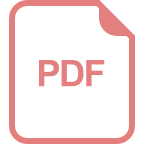
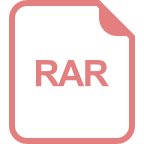











