pytorch画relu
时间: 2023-10-21 17:20:26 浏览: 88
PyTorch中的ReLU函数可以使用以下代码进行绘制:
```python
import torch
import matplotlib.pyplot as plt
x = torch.arange(-5., 5., 0.1)
y = torch.relu(x)
plt.plot(x.numpy(), y.numpy())
plt.show()
```
这段代码会生成一个以x为横轴,y为纵轴的ReLU函数图像。其中,torch.relu()函数是PyTorch中的ReLU函数,可以对输入进行ReLU操作。plt.plot()函数用于绘制图像,plt.show()函数用于显示图像。
相关问题
pytorch leakyrelu
PyTorch中的LeakyReLU是一种激活函数,它在输入小于零时引入一个小的负斜率,以便在负值区域保持一定的激活。这有助于解决传统ReLU函数在负值区域上的死亡梯度问题。
在PyTorch中,你可以使用`torch.nn.LeakyReLU`类来实现LeakyReLU激活函数。下面是一个简单的例子:
```python
import torch
import torch.nn as nn
leaky_relu = nn.LeakyReLU(negative_slope=0.2)
input_tensor = torch.randn(10) # 输入张量
output = leaky_relu(input_tensor) # 应用LeakyReLU激活函数
```
在上面的例子中,`negative_slope`参数指定了负斜率的值,通常取一个小于1的数。默认情况下,`negative_slope`的值为0.01。
希望这能够帮助到你!如果你还有其他问题,请随时问我。
pytorch的relu
PyTorch中的ReLU函数有两种实现方式:一种是torch.nn.ReLU(),另一种是torch.functional.relu()。其中,torch.nn.ReLU()是一个网络层,可以在模型中使用;而torch.functional.relu()是一个函数,可以在模型的forward方法中使用。
以下是两种实现方式的例子:
1.使用torch.nn.ReLU()实现ReLU函数:
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
return x
net = Net()
input = torch.randn(1, 10)
output = net(input)
print(output)
```
2.使用torch.functional.relu()实现ReLU函数:
```python
import torch
import torch.nn.functional as F
input = torch.randn(1, 10)
output = F.relu(input)
print(output)
```
阅读全文
相关推荐
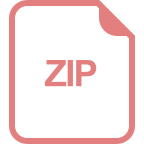




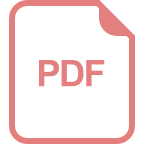









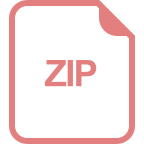