python 连接oracle库的代码
时间: 2023-02-21 10:40:50 浏览: 31
下面是连接 Oracle 数据库的 Python 代码示例,需要使用 cx_Oracle 库:
```
import cx_Oracle
# 连接字符串
dsn_tns = cx_Oracle.makedsn('hostname', 'port', service_name='servicename')
# 连接数据库
conn = cx_Oracle.connect(user='user', password='password', dsn=dsn_tns)
# 创建游标
cursor = conn.cursor()
# 执行查询
cursor.execute("SELECT * FROM table_name")
# 获取结果集
rows = cursor.fetchall()
# 遍历结果集
for row in rows:
print(row)
# 关闭游标
cursor.close()
# 关闭连接
conn.close()
```
请注意,您需要替换 `hostname`、`port`、`servicename`、`user` 和 `password` 的值。
相关问题
python 连接 oracle 代码
以下是一个简单的 Python 代码示例,用于连接 Oracle 数据库:
```python
import cx_Oracle
# 连接数据库
connection = cx_Oracle.connect('username/password@hostname:port/service_name')
# 创建游标对象
cursor = connection.cursor()
# 执行 SQL 查询
cursor.execute('SELECT * FROM table_name')
# 获取查询结果
for row in cursor:
print(row)
# 关闭游标和连接
cursor.close()
connection.close()
```
需要注意的是,在连接 Oracle 数据库时,需要提供以下信息:
- `username`:Oracle 数据库的用户名
- `password`:Oracle 数据库的密码
- `hostname`:Oracle 数据库的主机名或 IP 地址
- `port`:Oracle 数据库的端口号
- `service_name`:Oracle 数据库的服务名称
此外,还需要安装 `cx_Oracle` 模块,可以使用以下命令进行安装:
```
pip install cx_Oracle
```
python中oracle 库的连接代码
在Python中,连接Oracle数据库通常会使用cx_Oracle库,这是一个流行的Python绑定,它允许你通过Python与Oracle数据库进行交互。以下是基本的连接代码示例:
```python
import cx_Oracle
# 设置Oracle数据库连接的信息
dsn = cx_Oracle.makedsn('hostname', 'port', sid='service_name') # 替换为实际的主机名、端口号和SID
username = 'your_username'
password = 'your_password'
# 创建连接
connection = cx_Oracle.connect(username, password, dsn)
try:
# 创建游标对象,用于执行SQL语句
cursor = connection.cursor()
# 示例查询
cursor.execute("SELECT * FROM your_table")
# 获取查询结果
rows = cursor.fetchall()
for row in rows:
print(row)
finally:
# 关闭游标和连接
cursor.close()
connection.close()
```
在这个例子中,你需要替换`hostname`、`port`、`sid`、`your_username`和`your_password`为你的实际Oracle数据库的配置。注意,cx_Oracle库需要单独安装,可以使用pip命令安装:
```bash
pip install cx_Oracle
```
如果你打算长期保持连接打开,还可以考虑使用with语句来自动处理连接的关闭,例如:
```python
with cx_Oracle.connect(username, password, dsn) as connection:
cursor = connection.cursor()
# 执行其他操作...
```
这样可以确保在操作完成后,连接会被自动关闭。
阅读全文
相关推荐
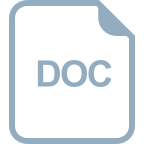
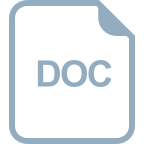
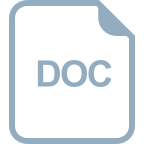


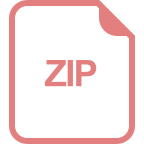






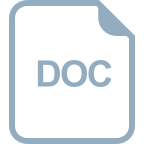
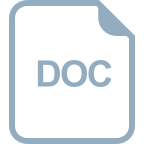
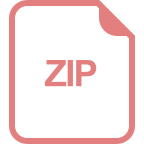