python连接oracle的库
时间: 2023-08-16 16:24:45 浏览: 56
在 Python 中连接 Oracle 数据库需要使用到 cx_Oracle 模块。下面是连接 Oracle 数据库的步骤:
1. 安装 cx_Oracle
可以使用 pip 命令安装 cx_Oracle:
```
pip install cx_Oracle
```
2. 导入 cx_Oracle 模块
在 Python 中使用 cx_Oracle,需要先导入该模块:
```python
import cx_Oracle
```
3. 连接 Oracle 数据库
使用 `cx_Oracle.connect()` 方法连接 Oracle 数据库,方法的参数中需要指定用户名、密码、主机名和 SID 等信息,例如:
```python
dsn = cx_Oracle.makedsn("localhost", "1521", "ORCL")
mydb = cx_Oracle.connect(user="username", password="password", dsn=dsn)
```
4. 创建游标对象
使用 `cursor()` 方法创建游标对象,该对象用于执行 SQL 语句:
```python
mycursor = mydb.cursor()
```
5. 执行 SQL 语句
使用游标对象的 `execute()` 方法执行 SQL 语句,例如:
```python
mycursor.execute("SELECT * FROM customers")
```
6. 获取查询结果
使用游标对象的 `fetchall()` 方法获取查询结果:
```python
result = mycursor.fetchall()
for row in result:
print(row)
```
完整的示例代码:
```python
import cx_Oracle
dsn = cx_Oracle.makedsn("localhost", "1521", "ORCL")
mydb = cx_Oracle.connect(user="username", password="password", dsn=dsn)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers")
result = mycursor.fetchall()
for row in result:
print(row)
```
相关推荐
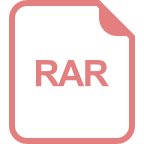
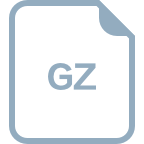
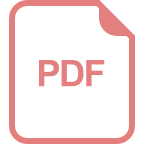














