python 多核并行计算
时间: 2023-08-08 08:12:23 浏览: 112
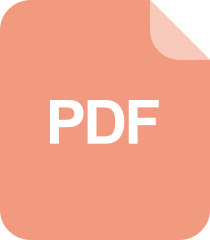
Python 多核并行计算的示例代码

Python中实现多核并行计算的方法有很多,下面介绍几种常用的方法:
1. 使用multiprocessing模块:multiprocessing模块是Python标准库中用于实现多进程编程的模块。通过创建多个进程来实现并行计算。可以使用Process类创建进程对象,并使用join()方法等待所有进程执行完毕。
2. 使用concurrent.futures模块:concurrent.futures模块是Python3中新增的模块,提供了高级别的接口来实现并行计算。可以使用ThreadPoolExecutor或ProcessPoolExecutor类来创建线程池或进程池,并使用submit()方法提交任务。
3. 使用joblib模块:joblib是一个用于并行计算的库,提供了Parallel函数和delayed装饰器,可以方便地实现并行计算。可以使用Parallel函数创建一个并行计算的上下文,并使用delayed装饰器包装需要并行执行的函数。
4. 使用mpi4py模块:mpi4py是Python的一个MPI(Message Passing Interface)并行计算库,可以在多个进程之间进行通信和协调。需要安装MPI库(如OpenMPI),然后使用mpiexec命令来执行Python脚本。
这些方法都可以用于实现Python的多核并行计算,具体选择哪种方法取决于你的需求和项目特点。
阅读全文
相关推荐


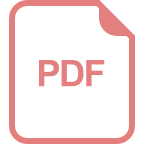
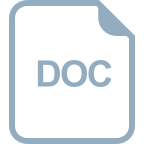
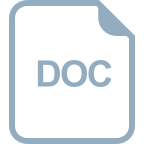
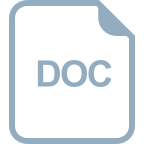
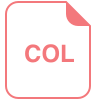
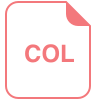
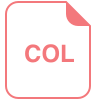
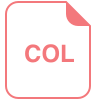
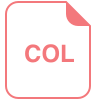
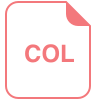
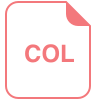
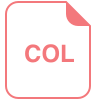
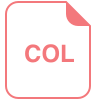