获取价格低于30的书名 # ********** begin ********* # print(tree.xpath('//book
时间: 2023-11-25 16:50:46 浏览: 63
以下是获取价格低于30的书名的代码示例:
```python
book_names = tree.xpath('//book[price<30]/title/text()')
print(book_names)
```
解释:
- `tree.xpath()`函数用于在HTML文档中选择元素。
- `//book[price<30]`表示选择价格小于30的所有book元素。
- `/title/text()`表示选择book元素下的title元素的文本内容。
- `book_names`变量存储了所有符合条件的书名。
- `print(book_names)`输出所有符合条件的书名。
相关问题
填写代码, 获取价格低于30的书名 # ********** Begin ********* # print(tree.xpath('')) # *********** End ********** #
要获取价格低于30的书名,可以使用XPath来筛选符合条件的节点。以下是一个示例代码:
# ********** Begin ********* #
book_names = tree.xpath('//book[price < 30]/title/text()')
print(book_names)
# *********** End ********** #
这段代码使用XPath表达式`//book[price < 30]/title/text()`来选择价格低于30的书籍节点,并获取它们的标题文本。最后将书名打印出来。
用c++写图书期刊信息管理系统 阅览室对所收藏的图书与期刊信息实现计算机管理,为用户提供图书信息的查询。阅览室陈列若干工具书、期刊和其他书籍供读者在阅览室内查阅。同一刊物可以有去年和当年的多期同时放在书架上。阅览室的图书信息使用文件保存,按照书名排序。有新书刊到达时,工作人员将新书的信息添加到文件中。书籍的信息包括书刊编号、书名、出版单位、出版时间、价格、书籍分类号等,其中,期刊的书名包括刊物名称与年份期数,例如:读者-2014-21。每年的7月份开始,上一年的期刊将陆续从书架上撤走,装订成册,变成合订本上架,同时,图书信息文件中要刑去这些已经撤走的过期期刊的信息,添加合订本期刊的信息,例如,书名为读者-2014合。 图书期刊信息管理系统提供对书籍信息的录入与删除功能,提供图书信息的浏览与查询功能。其中。查询可以是对书名、出版单位、书籍分类号的模糊 查询。
下面是一个简单的C++程序,实现了图书期刊信息管理系统的基本功能:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
struct Book {
string id;
string name;
string publisher;
string publish_time;
double price;
string category;
};
struct Journal {
string id;
string name;
string year;
int issue;
string publisher;
string publish_time;
double price;
string category;
};
vector<Book> books;
vector<Journal> journals;
void load_data() {
ifstream book_file("book.txt");
if (book_file) {
Book book;
while (book_file >> book.id >> book.name >> book.publisher >> book.publish_time >> book.price >> book.category) {
books.push_back(book);
}
book_file.close();
}
ifstream journal_file("journal.txt");
if (journal_file) {
Journal journal;
while (journal_file >> journal.id >> journal.name >> journal.year >> journal.issue >> journal.publisher >> journal.publish_time >> journal.price >> journal.category) {
journals.push_back(journal);
}
journal_file.close();
}
}
void save_data() {
ofstream book_file("book.txt");
if (book_file) {
for (const auto& book : books) {
book_file << book.id << " " << book.name << " " << book.publisher << " " << book.publish_time << " " << book.price << " " << book.category << endl;
}
book_file.close();
}
ofstream journal_file("journal.txt");
if (journal_file) {
for (const auto& journal : journals) {
journal_file << journal.id << " " << journal.name << " " << journal.year << " " << journal.issue << " " << journal.publisher << " " << journal.publish_time << " " << journal.price << " " << journal.category << endl;
}
journal_file.close();
}
}
void add_book() {
Book book;
cout << "请输入书籍编号:";
cin >> book.id;
cout << "请输入书名:";
cin >> book.name;
cout << "请输入出版单位:";
cin >> book.publisher;
cout << "请输入出版时间:";
cin >> book.publish_time;
cout << "请输入价格:";
cin >> book.price;
cout << "请输入书籍分类号:";
cin >> book.category;
books.push_back(book);
cout << "添加成功!" << endl;
}
void add_journal() {
Journal journal;
cout << "请输入期刊编号:";
cin >> journal.id;
cout << "请输入期刊名称:";
cin >> journal.name;
cout << "请输入年份:";
cin >> journal.year;
cout << "请输入期数:";
cin >> journal.issue;
cout << "请输入出版单位:";
cin >> journal.publisher;
cout << "请输入出版时间:";
cin >> journal.publish_time;
cout << "请输入价格:";
cin >> journal.price;
cout << "请输入期刊分类号:";
cin >> journal.category;
journals.push_back(journal);
cout << "添加成功!" << endl;
}
void remove_book() {
string id;
cout << "请输入要删除的书籍编号:";
cin >> id;
auto it = find_if(books.begin(), books.end(), [id](const Book& book) { return book.id == id; });
if (it != books.end()) {
books.erase(it);
cout << "删除成功!" << endl;
} else {
cout << "未找到该书籍!" << endl;
}
}
void remove_journal() {
string id;
cout << "请输入要删除的期刊编号:";
cin >> id;
auto it = find_if(journals.begin(), journals.end(), [id](const Journal& journal) { return journal.id == id; });
if (it != journals.end()) {
journals.erase(it);
cout << "删除成功!" << endl;
} else {
cout << "未找到该期刊!" << endl;
}
}
void print_book(const Book& book) {
cout << book.id << " " << book.name << " " << book.publisher << " " << book.publish_time << " " << book.price << " " << book.category << endl;
}
void print_journal(const Journal& journal) {
cout << journal.id << " " << journal.name << " " << journal.year << " " << journal.issue << " " << journal.publisher << " " << journal.publish_time << " " << journal.price << " " << journal.category << endl;
}
void browse_book() {
cout << "书名\t出版单位\t出版时间\t价格\t书籍分类号" << endl;
for (const auto& book : books) {
print_book(book);
}
}
void browse_journal() {
cout << "期刊名称\t年份\t期数\t出版单位\t出版时间\t价格\t期刊分类号" << endl;
for (const auto& journal : journals) {
print_journal(journal);
}
}
void query_book() {
string keyword;
cout << "请输入查询关键词:";
cin >> keyword;
cout << "书名\t出版单位\t出版时间\t价格\t书籍分类号" << endl;
for (const auto& book : books) {
if (book.name.find(keyword) != string::npos || book.publisher.find(keyword) != string::npos || book.category.find(keyword) != string::npos) {
print_book(book);
}
}
}
void query_journal() {
string keyword;
cout << "请输入查询关键词:";
cin >> keyword;
cout << "期刊名称\t年份\t期数\t出版单位\t出版时间\t价格\t期刊分类号" << endl;
for (const auto& journal : journals) {
if (journal.name.find(keyword) != string::npos || journal.publisher.find(keyword) != string::npos || journal.category.find(keyword) != string::npos) {
print_journal(journal);
}
}
}
void update_journal() {
string year;
cout << "请输入要更新的年份:";
cin >> year;
string name = year + "-";
cout << "请输入要更新的期刊名称:";
cin >> name;
auto it = find_if(journals.begin(), journals.end(), [name](const Journal& journal) { return journal.name == name; });
if (it != journals.end()) {
for (auto& journal : journals) {
if (journal.year == year && journal.name.find(name) != string::npos) {
journal.name = name + "合";
}
}
cout << "更新成功!" << endl;
} else {
cout << "未找到该期刊!" << endl;
}
}
int main() {
load_data();
int choice;
do {
cout << "欢迎使用图书期刊信息管理系统!" << endl;
cout << "1. 添加书籍" << endl;
cout << "2. 添加期刊" << endl;
cout << "3. 删除书籍" << endl;
cout << "4. 删除期刊" << endl;
cout << "5. 浏览书籍" << endl;
cout << "6. 浏览期刊" << endl;
cout << "7. 查询书籍" << endl;
cout << "8. 查询期刊" << endl;
cout << "9. 更新期刊" << endl;
cout << "0. 退出系统" << endl;
cout << "请选择操作:";
cin >> choice;
switch (choice) {
case 1:
add_book();
save_data();
break;
case 2:
add_journal();
save_data();
break;
case 3:
remove_book();
save_data();
break;
case 4:
remove_journal();
save_data();
break;
case 5:
browse_book();
break;
case 6:
browse_journal();
break;
case 7:
query_book();
break;
case 8:
query_journal();
break;
case 9:
update_journal();
save_data();
break;
case 0:
break;
default:
cout << "无效的操作!" << endl;
break;
}
} while (choice != 0);
return 0;
}
```
程序中使用了两个结构体 `Book` 和 `Journal`,分别表示书籍和期刊的信息。程序通过文件读写实现了对图书信息的持久化管理。程序中实现了添加、删除、浏览和查询等功能,其中查询支持模糊查询。另外,程序还实现了更新期刊的功能,以应对每年撤销过期期刊的情况。
阅读全文
相关推荐
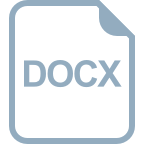
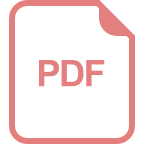











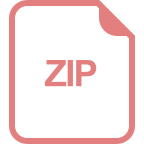
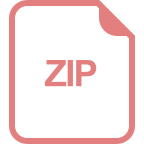