基于c++设计一个书店管理系统,能完成书店的日常管理工作。 要求完成的基本功能: 1、进货入库记录。 2、销售出货记录。 3、图书信息查询:可通过书名、作者等途径查询某本图书的详细信息(含书名、作者、出版社、页数、最新入库时间、库存量、价格等)。 4、自动预警提示(当某图书的库存量为1时自动预警提示) 5、数据存储:以文件方式存储出入库记录 可自行补充其它功能。
时间: 2023-07-10 16:13:41 浏览: 93
以下是一个简单的书店管理系统的 C++ 实现,包含了题目要求的基本功能:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
#include <ctime>
using namespace std;
// 书籍类
class Book {
private:
string name;
string author;
string publisher;
int page;
double price;
int stock;
time_t latest_stock_time;
public:
Book(string n, string a, string p, int pg, double pr, int s) {
name = n;
author = a;
publisher = p;
page = pg;
price = pr;
stock = s;
latest_stock_time = time(nullptr);
}
// 获取书名
string getName() const { return name; }
// 获取作者
string getAuthor() const { return author; }
// 获取出版社
string getPublisher() const { return publisher; }
// 获取页数
int getPage() const { return page; }
// 获取价格
double getPrice() const { return price; }
// 获取库存量
int getStock() const { return stock; }
// 获取最新入库时间
time_t getLatestStockTime() const { return latest_stock_time; }
// 设置库存量
void setStock(int s) {
stock = s;
latest_stock_time = time(nullptr);
}
// 打印书籍信息
void print() const {
cout << "书名:" << name << endl;
cout << "作者:" << author << endl;
cout << "出版社:" << publisher << endl;
cout << "页数:" << page << endl;
cout << "价格:" << price << endl;
cout << "库存量:" << stock << endl;
cout << "最新入库时间:" << ctime(&latest_stock_time) << endl;
}
};
// 书店类
class Bookstore {
private:
vector<Book> books; // 存储所有书籍信息的容器
string record_file; // 存储出入库记录的文件名
public:
Bookstore(string rf) {
record_file = rf;
readRecordFile(); // 读取出入库记录文件
}
// 进货入库记录
void purchase(string name, int num) {
auto iter = find_if(books.begin(), books.end(),
[&](const Book& b) { return b.getName() == name; });
if (iter != books.end()) {
iter->setStock(iter->getStock() + num);
} else {
string author, publisher;
int page;
double price;
cout << "请输入书籍作者:" << endl;
getline(cin, author);
cout << "请输入书籍出版社:" << endl;
getline(cin, publisher);
cout << "请输入书籍页数:" << endl;
cin >> page;
cout << "请输入书籍价格:" << endl;
cin >> price;
cin.ignore();
books.emplace_back(name, author, publisher, page, price, num);
}
writeRecordFile("进货", name, num);
cout << "进货成功!" << endl;
}
// 销售出货记录
void sell(string name, int num) {
auto iter = find_if(books.begin(), books.end(),
[&](const Book& b) { return b.getName() == name; });
if (iter == books.end()) {
cout << "没有找到该书籍!" << endl;
return;
}
if (iter->getStock() < num) {
cout << "库存不足!" << endl;
return;
}
iter->setStock(iter->getStock() - num);
writeRecordFile("销售", name, num);
cout << "销售成功!" << endl;
}
// 图书信息查询
void search(string name) const {
auto iter = find_if(books.begin(), books.end(),
[&](const Book& b) { return b.getName() == name; });
if (iter == books.end()) {
cout << "没有找到该书籍!" << endl;
return;
}
iter->print();
}
// 自动预警提示
void alert() const {
for (const auto& book : books) {
if (book.getStock() == 1) {
cout << "书籍 " << book.getName() << " 库存不足,请及时补货!" << endl;
}
}
}
// 读取出入库记录文件
void readRecordFile() {
ifstream fin(record_file);
if (!fin) return;
string type, name;
int num;
while (fin >> type >> name >> num) {
if (type == "进货") {
purchase(name, num);
} else if (type == "销售") {
sell(name, num);
}
}
}
// 写入出入库记录文件
void writeRecordFile(string type, string name, int num) const {
ofstream fout(record_file, ios::app);
fout << type << " " << name << " " << num << endl;
}
};
int main() {
Bookstore bookstore("record.txt");
int choice;
while (true) {
cout << "请选择操作:1.进货 2.销售 3.书籍信息查询 4.预警提示 5.退出" << endl;
cin >> choice;
cin.ignore();
switch (choice) {
case 1: {
string name;
int num;
cout << "请输入书籍名称:" << endl;
getline(cin, name);
cout << "请输入进货数量:" << endl;
cin >> num;
cin.ignore();
bookstore.purchase(name, num);
break;
}
case 2: {
string name;
int num;
cout << "请输入书籍名称:" << endl;
getline(cin, name);
cout << "请输入销售数量:" << endl;
cin >> num;
cin.ignore();
bookstore.sell(name, num);
break;
}
case 3: {
string name;
cout << "请输入书籍名称:" << endl;
getline(cin, name);
bookstore.search(name);
break;
}
case 4: {
bookstore.alert();
break;
}
case 5:
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
这个程序中,书籍信息使用 `Book` 类来表示,包含了书名、作者、出版社、页数、价格、库存量和最新入库时间等属性。`Bookstore` 类是书店类,包含了所有书籍信息的容器 `books` 和存储出入库记录的文件名 `record_file`。书店类实现了进货、销售、图书信息查询和自动预警提示等功能,同时还可以将出入库记录存储到文件中。
在 `main()` 函数中,使用一个无限循环来不断接受用户的操作选择,并根据选择调用相应的函数来完成操作。
阅读全文
相关推荐
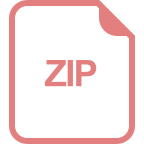
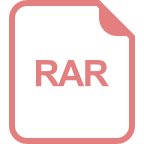

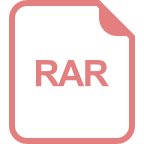
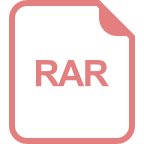
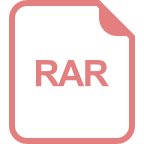
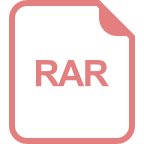
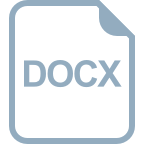
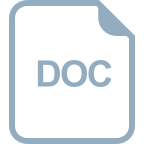







