使用c++,运用class和vector的知识,写出一个书店管理系统的代码,要求完成的基本功能: 1、进货入库记录。 2、销售出货记录。 3、图书信息查询:可通过书名、作者等途径查询某本图书的详细信息(含书名、作者、出版社、页数、最新入库时间、库存量、价格等)。 4、自动预警提示(当某图书的库存量为1时自动预警提示) 5、数据存储:以文件方式存储出入库记录
时间: 2023-07-04 14:12:57 浏览: 59
以下是一个简单的书店管理系统的代码示例,但需要读者自行完善和测试。该系统使用了class和vector来管理图书信息,并使用文件存储出入库记录。
```c++
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
using namespace std;
// 定义图书信息类
class BookInfo {
public:
BookInfo(string title, string author, string publisher, int pages, int stock, double price) {
this->title = title;
this->author = author;
this->publisher = publisher;
this->pages = pages;
this->stock = stock;
this->price = price;
}
// 成员函数:获取图书信息
string getTitle() {
return title;
}
string getAuthor() {
return author;
}
string getPublisher() {
return publisher;
}
int getPages() {
return pages;
}
int getStock() {
return stock;
}
double getPrice() {
return price;
}
// 成员函数:更新图书库存
void updateStock(int num) {
stock += num;
}
private:
string title; // 标题
string author; // 作者
string publisher; // 出版社
int pages; // 页数
int stock; // 库存量
double price; // 价格
};
// 定义进出库记录类
class Record {
public:
Record(string title, string type, int num) {
this->title = title;
this->type = type;
this->num = num;
}
// 成员函数:获取记录信息
string getTitle() {
return title;
}
string getType() {
return type;
}
int getNum() {
return num;
}
private:
string title; // 图书标题
string type; // 记录类型,入库或出库
int num; // 数量
};
// 定义书店管理系统类
class BookStore {
public:
// 成员函数:添加新书
void addBook(string title, string author, string publisher, int pages, int stock, double price) {
BookInfo book(title, author, publisher, pages, stock, price);
books.push_back(book);
}
// 成员函数:进货入库
void stockIn(string title, int num) {
for (int i = 0; i < books.size(); i++) {
if (books[i].getTitle() == title) {
books[i].updateStock(num);
Record record(title, "入库", num);
records.push_back(record);
cout << "进货入库成功!" << endl;
return;
}
}
cout << "未找到该书!" << endl;
}
// 成员函数:销售出货
void sellOut(string title, int num) {
for (int i = 0; i < books.size(); i++) {
if (books[i].getTitle() == title) {
if (books[i].getStock() >= num) {
books[i].updateStock(-num);
Record record(title, "出库", num);
records.push_back(record);
cout << "销售出货成功!" << endl;
} else {
cout << "库存不足!" << endl;
}
return;
}
}
cout << "未找到该书!" << endl;
}
// 成员函数:查询图书信息
void queryBook(string title) {
for (int i = 0; i < books.size(); i++) {
if (books[i].getTitle() == title) {
cout << "书名:" << books[i].getTitle() << endl;
cout << "作者:" << books[i].getAuthor() << endl;
cout << "出版社:" << books[i].getPublisher() << endl;
cout << "页数:" << books[i].getPages() << endl;
cout << "库存量:" << books[i].getStock() << endl;
cout << "价格:" << books[i].getPrice() << endl;
return;
}
}
cout << "未找到该书!" << endl;
}
// 成员函数:自动预警提示
void autoAlert() {
for (int i = 0; i < books.size(); i++) {
if (books[i].getStock() == 1) {
cout << books[i].getTitle() << " 库存量为1,请及时补充库存!" << endl;
}
}
}
// 成员函数:保存数据到文件
void saveToFile(string filename) {
ofstream outfile(filename);
if (outfile.is_open()) {
for (int i = 0; i < records.size(); i++) {
outfile << records[i].getTitle() << "\t" << records[i].getType() << "\t" << records[i].getNum() << endl;
}
outfile.close();
cout << "数据已保存到文件!" << endl;
} else {
cout << "无法打开文件!" << endl;
}
}
private:
vector<BookInfo> books; // 图书信息列表
vector<Record> records; // 进出库记录列表
};
int main() {
BookStore store;
store.addBook("C++ Primer", "Lippman", "O'Reilly", 1376, 10, 99.0);
store.addBook("Effective C++", "Meyers", "Addison-Wesley", 320, 5, 49.99);
store.stockIn("C++ Primer", 5);
store.sellOut("Effective C++", 3);
store.queryBook("C++ Primer");
store.autoAlert();
store.saveToFile("records.txt");
return 0;
}
```
注意:以上代码只是一个简单的示例,可能存在一些潜在的问题和不足之处。读者需要自行完善和测试,确保代码的正确性和可靠性。
相关推荐
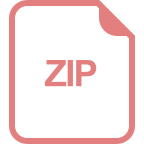
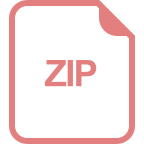
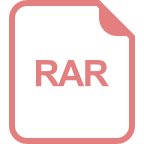














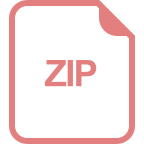