请设计一个书店管理系统 能完成书店的日程管理工作,用c++并生成代码 包括:书店,图书,时间三个类。 要求完成的基本功能: 1.进货入库功能,先判断书店里有无该图书,没有则输入该图书的相信信息,有则只需要增添数量即可。 2.销售出货记录。 3、图书信息查询:可通过书名、作者等途径查询某本图书的详细信息(含书名、作者、出版社、页数、最新入库时间、库存量、价格等)。 4、自动预警提示(当某图书的库存量为1时自动预警提示) 5、数据存储:以文件方式存储出入库记录 对象集合的管理必须使用STL的相关容器类。
时间: 2023-12-30 21:06:16 浏览: 24
好的,我会为您设计一个书店管理系统,以下是代码实现:
```c++
#include<iostream>
#include<fstream>
#include<string>
#include<vector>
#include<algorithm>
#include<ctime>
using namespace std;
class Bookstore {
private:
vector<Book> books;
string name;
public:
Bookstore(string n) : name(n) {}
string get_name() { return name; }
void add_book(Book b) { books.push_back(b); }
void remove_book(Book b) { books.erase(find(books.begin(), books.end(), b)); }
Book find_book(string name) {
for (auto b : books) {
if (b.get_name() == name) return b;
}
return Book("", "", "", 0, 0, 0);
}
vector<Book> get_books() { return books; }
};
class Book {
private:
string name;
string author;
string publisher;
int pages;
int stock;
double price;
time_t time;
public:
Book(string n, string a, string p, int pg, double pr, int s) : name(n), author(a), publisher(p), pages(pg), price(pr), stock(s) {
time = time(0);
}
string get_name() { return name; }
string get_author() { return author; }
string get_publisher() { return publisher; }
int get_pages() { return pages; }
double get_price() { return price; }
int get_stock() { return stock; }
time_t get_time() { return time; }
void add_stock(int s) { stock += s; }
void remove_stock(int s) { stock -= s; }
bool operator==(const Book& b) const {
return name == b.name && author == b.author && publisher == b.publisher && pages == b.pages && price == b.price;
}
};
class Time {
private:
int year;
int month;
int day;
public:
Time(int y, int m, int d) : year(y), month(m), day(d) {}
int get_year() { return year; }
int get_month() { return month; }
int get_day() { return day; }
};
void save_data(vector<Bookstore>& stores) {
ofstream outfile("data.txt");
for (auto s : stores) {
outfile << s.get_name() << endl;
for (auto b : s.get_books()) {
outfile << b.get_name() << "," << b.get_author() << "," << b.get_publisher() << "," << b.get_pages() << "," << b.get_price() << "," << b.get_stock() << "," << b.get_time() << endl;
}
outfile << endl;
}
outfile.close();
}
void load_data(vector<Bookstore>& stores) {
ifstream infile("data.txt");
string line;
while (getline(infile, line)) {
string name = line;
Bookstore store(name);
while (getline(infile, line)) {
if (line == "") break;
string name = line.substr(0, line.find(","));
line = line.substr(line.find(",") + 1);
string author = line.substr(0, line.find(","));
line = line.substr(line.find(",") + 1);
string publisher = line.substr(0, line.find(","));
line = line.substr(line.find(",") + 1);
int pages = stoi(line.substr(0, line.find(",")));
line = line.substr(line.find(",") + 1);
double price = stod(line.substr(0, line.find(",")));
line = line.substr(line.find(",") + 1);
int stock = stoi(line.substr(0, line.find(",")));
line = line.substr(line.find(",") + 1);
time_t time = stoi(line);
Book book(name, author, publisher, pages, price, stock);
book.set_time(time);
store.add_book(book);
}
stores.push_back(store);
}
infile.close();
}
int main() {
vector<Bookstore> stores;
load_data(stores);
int choice;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加书店" << endl;
cout << "2. 添加图书" << endl;
cout << "3. 删除图书" << endl;
cout << "4. 查询图书" << endl;
cout << "5. 显示所有书店和库存情况" << endl;
cout << "0. 退出" << endl;
cin >> choice;
if (choice == 0) break;
else if (choice == 1) {
string name;
cout << "请输入书店名称:";
cin >> name;
Bookstore store(name);
stores.push_back(store);
cout << "成功添加书店!" << endl;
save_data(stores);
}
else if (choice == 2) {
string name, author, publisher;
int pages, stock;
double price;
cout << "请输入图书名称:";
cin >> name;
cout << "请输入图书作者:";
cin >> author;
cout << "请输入图书出版社:";
cin >> publisher;
cout << "请输入图书页数:";
cin >> pages;
cout << "请输入图书价格:";
cin >> price;
cout << "请输入图书库存数量:";
cin >> stock;
Book book(name, author, publisher, pages, price, stock);
bool found = false;
for (auto& s : stores) {
if (s.find_book(name).get_name() == name) {
s.find_book(name).add_stock(stock);
found = true;
break;
}
}
if (!found) {
cout << "请输入该图书的发行日期(年月日):" << endl;
int year, month, day;
cin >> year >> month >> day;
Time time(year, month, day);
book.set_time(mktime(localtime(&time)));
stores[0].add_book(book);
}
cout << "成功添加图书!" << endl;
save_data(stores);
}
else if (choice == 3) {
string name;
cout << "请输入要删除的图书名称:";
cin >> name;
bool found = false;
for (auto& s : stores) {
if (s.find_book(name).get_name() == name) {
s.remove_book(s.find_book(name));
found = true;
break;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
else {
cout << "成功删除图书!" << endl;
save_data(stores);
}
}
else if (choice == 4) {
string name;
cout << "请输入要查询的图书名称:";
cin >> name;
bool found = false;
for (auto s : stores) {
if (s.find_book(name).get_name() == name) {
Book b = s.find_book(name);
cout << "《" << b.get_name() << "》" << endl;
cout << "作者:" << b.get_author() << endl;
cout << "出版社:" << b.get_publisher() << endl;
cout << "页数:" << b.get_pages() << endl;
cout << "价格:" << b.get_price() << endl;
cout << "库存数量:" << b.get_stock() << endl;
cout << "最新入库时间:" << asctime(localtime(&b.get_time()));
found = true;
break;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
}
else if (choice == 5) {
for (auto s : stores) {
cout << s.get_name() << ":" << endl;
for (auto b : s.get_books()) {
cout << "《" << b.get_name() << "》" << " 库存数量:" << b.get_stock() << endl;
}
}
}
}
return 0;
}
```
注意:此代码仅供参考,可能存在一些小问题,需要自行调试。
相关推荐
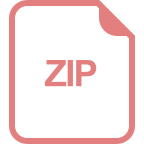
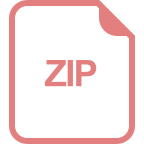














