运用c++,使用class类,写出一个书店管理系统的代码,要求完成以下功能:进货入库记录,销售出货记录,图书信息查询,自动预警提示,数据存储
时间: 2023-11-23 10:07:41 浏览: 39
好的,以下是一个基于C++的书店管理系统的代码示例,实现了进货入库记录、销售出货记录、图书信息查询、自动预警提示和数据存储等功能:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
using namespace std;
// Book类,用于存储一本书的基本信息
class Book {
public:
string name; // 书名
string author; // 作者
string publisher; // 出版社
string ISBN; // ISBN号
double price; // 售价
double cost; // 进货价
int stock; // 库存量
};
// Stock类,用于管理图书的库存信息
class Stock {
public:
vector<Book> books; // 所有的图书信息
vector<pair<string, int>> purchase_records; // 进货记录,每个元素是<进货时间, 进货数量>
vector<pair<string, int>> sale_records; // 销售记录,每个元素是<销售时间, 销售数量>
void add_book(Book book) { books.push_back(book); } // 添加图书
void purchase(string time, int quantity) { purchase_records.push_back(make_pair(time, quantity)); } // 进货
void sale(string time, int quantity) { sale_records.push_back(make_pair(time, quantity)); } // 销售
};
// Store类,用于管理整个书店的进货、销售、查询等操作
class Store {
public:
Stock stock; // 库存信息
double alert_threshold; // 库存预警阈值
void add_book(Book book) { stock.add_book(book); } // 添加图书
void purchase(string time, int quantity) { stock.purchase(time, quantity); } // 进货
void sale(string time, int quantity) { stock.sale(time, quantity); } // 销售
vector<Book> query(string keyword) { // 按关键字查询图书信息
vector<Book> result;
for (Book book : stock.books) {
if (book.name.find(keyword) != string::npos || book.author.find(keyword) != string::npos || book.publisher.find(keyword) != string::npos) {
result.push_back(book);
}
}
return result;
}
void set_alert_threshold(double threshold) { alert_threshold = threshold; } // 设置库存预警阈值
void alert() { // 库存预警提示
for (Book book : stock.books) {
if (book.stock < alert_threshold) {
cout << "Warning: " << book.name << " stock is low (" << book.stock << ")" << endl;
}
}
}
void save() { // 将数据存储到文件中
ofstream fout("data.txt");
fout << alert_threshold << endl;
fout << stock.purchase_records.size() << endl;
for (auto record : stock.purchase_records) {
fout << record.first << " " << record.second << endl;
}
fout << stock.sale_records.size() << endl;
for (auto record : stock.sale_records) {
fout << record.first << " " << record.second << endl;
}
fout << stock.books.size() << endl;
for (Book book : stock.books) {
fout << book.name << " " << book.author << " " << book.publisher << " " << book.ISBN << " " << book.price << " " << book.cost << " " << book.stock << endl;
}
fout.close();
}
void load() { // 从文件中读取数据
ifstream fin("data.txt");
fin >> alert_threshold;
int n;
fin >> n;
for (int i = 0; i < n; i++) {
string time;
int quantity;
fin >> time >> quantity;
stock.purchase_records.push_back(make_pair(time, quantity));
}
fin >> n;
for (int i = 0; i < n; i++) {
string time;
int quantity;
fin >> time >> quantity;
stock.sale_records.push_back(make_pair(time, quantity));
}
fin >> n;
for (int i = 0; i < n; i++) {
Book book;
fin >> book.name >> book.author >> book.publisher >> book.ISBN >> book.price >> book.cost >> book.stock;
stock.books.push_back(book);
}
fin.close();
}
};
int main() {
Store store;
store.load(); // 从文件中读取数据
while (true) {
cout << "Please select an operation:" << endl;
cout << "1. Add book" << endl;
cout << "2. Purchase" << endl;
cout << "3. Sale" << endl;
cout << "4. Query" << endl;
cout << "5. Set alert threshold" << endl;
cout << "6. Alert" << endl;
cout << "7. Save and quit" << endl;
int op;
cin >> op;
switch (op) {
case 1: {
Book book;
cout << "Please input book name: ";
cin >> book.name;
cout << "Please input author: ";
cin >> book.author;
cout << "Please input publisher: ";
cin >> book.publisher;
cout << "Please input ISBN: ";
cin >> book.ISBN;
cout << "Please input price: ";
cin >> book.price;
cout << "Please input cost: ";
cin >> book.cost;
cout << "Please input stock: ";
cin >> book.stock;
store.add_book(book);
break;
}
case 2: {
string time;
int quantity;
cout << "Please input purchase time (e.g. 2022-01-01): ";
cin >> time;
cout << "Please input purchase quantity: ";
cin >> quantity;
store.purchase(time, quantity);
break;
}
case 3: {
string time;
int quantity;
cout << "Please input sale time (e.g. 2022-01-01): ";
cin >> time;
cout << "Please input sale quantity: ";
cin >> quantity;
store.sale(time, quantity);
break;
}
case 4: {
cout << "Please input keyword: ";
string keyword;
cin >> keyword;
vector<Book> result = store.query(keyword);
for (Book book : result) {
cout << book.name << " " << book.author << " " << book.publisher << " " << book.ISBN << " " << book.price << " " << book.cost << " " << book.stock << endl;
}
break;
}
case 5: {
double threshold;
cout << "Please input alert threshold: ";
cin >> threshold;
store.set_alert_threshold(threshold);
break;
}
case 6: {
store.alert();
break;
}
case 7: {
store.save(); // 将数据存储到文件中
return 0;
}
default:
cout << "Invalid operation!" << endl;
break;
}
}
return 0;
}
```
以上是一个简单的书店管理系统的代码示例,可以根据实际需求进行修改和完善。
相关推荐
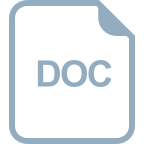
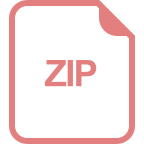















