STM8 以定时中断来做433遥控接收与解码程序
时间: 2024-04-16 07:26:14 浏览: 9
使用STM8的定时中断来实现433MHz遥控收与解码是一种常见的方法。下面是一个简单的示例程序,演示了如何使用STM8的定时器和外部中断来实现这一功能:
```c
#include <stm8s.h>
#define RF_PIN GPIO_PIN_1
#define RF_PORT GPIOC
volatile uint8_t receivedData = 0;
volatile uint8_t dataReady = 0;
void TIM1_UPD_OVF_TRG_COM_IRQHandler(void) __interrupt(11)
{
if (TIM1_GetITStatus(TIM1_IT_UPDATE) != RESET)
{
receivedData = 0;
TIM1_ClearITPendingBit(TIM1_IT_UPDATE);
}
}
void EXTI_PORTC_IRQHandler(void) __interrupt(5)
{
if (GPIO_ReadInputPin(RF_PORT, RF_PIN) == RESET)
{
TIM1_Cmd(DISABLE);
receivedData = 0;
dataReady = 0;
EXTI->SR1 |= GPIO_PIN_1; // Clear interrupt flag
}
else
{
TIM1_SetCounter(0);
TIM1_Cmd(ENABLE);
EXTI->SR1 |= GPIO_PIN_1; // Clear interrupt flag
}
}
void main(void)
{
CLK_HSIPrescalerConfig(CLK_PRESCALER_HSIDIV1); // Set CPU clock frequency
TIM1_TimeBaseInit(0, TIM1_COUNTERMODE_UP, 30000, 0); // Set timer period, adjust as needed
TIM1_ITConfig(TIM1_IT_UPDATE, ENABLE); // Enable timer interrupt
GPIO_Init(RF_PORT, RF_PIN, GPIO_MODE_IN_FL_IT); // Configure RF pin as input with interrupt
EXTI_SetExtIntSensitivity(EXTI_PORT_GPIOC, EXTI_SENSITIVITY_RISE_FALL); // Trigger interrupt on both rising and falling edges
enableInterrupts(); // Enable global interrupts
while (1)
{
if (dataReady)
{
// Do something with received data
// ...
dataReady = 0;
}
}
}
```
在这个示例程序中,我们使用STM8的定时器(Timer 1)和外部中断来实现定时中断功能。当接收到无线信号时,外部中断触发并启动定时器,定时器定时一段时间后触发定时中断。在定时中断中,我们可以根据定时器计数值判断信号的高低电平持续时间,并解码接收到的信号。如果解码成功,我们可以在主循环中对接收到的数据进行处理。
需要注意的是,定时器的计数值和定时中断的触发频率需要根据具体的应用需求和硬件配置进行调整。此示例程序仅提供了一个基本的框架,实际的实现可能需要根据具体情况进行优化和调整。
相关推荐
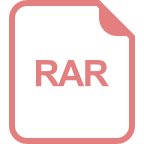
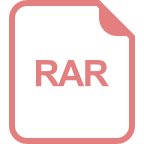














