用java 写一个斗地主程序
时间: 2023-10-31 08:04:12 浏览: 43
好的,以下是一个简单的斗地主程序示例,仅供参考:
```java
import java.util.*;
public class DouDiZhuGame {
public static void main(String[] args) {
// 初始化牌堆
List<String> poker = initPoker();
// 洗牌
Collections.shuffle(poker);
// 初始化玩家
Player player1 = new Player("张三");
Player player2 = new Player("李四");
Player player3 = new Player("王五");
// 发牌
dealPoker(poker, player1, player2, player3);
// 叫地主
Player landlord = callLandlord(player1, player2, player3);
// 出牌
playPoker(landlord, player1, player2, player3);
// 计算得分
calculateScore(player1, player2, player3, landlord);
}
// 初始化牌堆
public static List<String> initPoker() {
List<String> poker = new ArrayList<>();
String[] colors = {"♠", "♥", "♣", "♦"};
String[] numbers = {"3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A", "2"};
for (String color : colors) {
for (String number : numbers) {
poker.add(color + number);
}
}
poker.add("🃏");
poker.add("👑");
return poker;
}
// 发牌
public static void dealPoker(List<String> poker, Player player1, Player player2, Player player3) {
for (int i = 0; i < poker.size(); i++) {
String card = poker.get(i);
if (i % 3 == 0) {
player1.addCard(card);
} else if (i % 3 == 1) {
player2.addCard(card);
} else {
player3.addCard(card);
}
}
}
// 叫地主
public static Player callLandlord(Player player1, Player player2, Player player3) {
int maxScore = 0;
Player landlord = null;
Scanner scanner = new Scanner(System.in);
for (Player player : new Player[]{player1, player2, player3}) {
System.out.println(player.getName() + "叫地主,输入叫分(0-3),0表示不叫:");
int score = scanner.nextInt();
if (score > maxScore) {
maxScore = score;
landlord = player;
}
player.setScore(score);
}
System.out.println(landlord.getName() + "成为地主!");
return landlord;
}
// 出牌
public static void playPoker(Player landlord, Player player1, Player player2, Player player3) {
List<String> poker = new ArrayList<>();
poker.addAll(landlord.getCards());
Scanner scanner = new Scanner(System.in);
while (true) {
Player currentPlayer = null;
if (poker.isEmpty()) {
currentPlayer = landlord;
} else {
currentPlayer = getNextPlayer(player1, player2, player3, landlord);
}
System.out.println(currentPlayer.getName() + "出牌,请输入牌(多张牌用空格隔开):");
String[] cards = scanner.nextLine().split(" ");
List<String> playCards = Arrays.asList(cards);
if (!currentPlayer.checkCards(playCards)) {
System.out.println("出牌不符合规则,请重新出牌!");
continue;
}
currentPlayer.removeCards(playCards);
poker = playCards;
System.out.println(currentPlayer.getName() + "出牌:" + poker);
if (currentPlayer.getCards().isEmpty()) {
System.out.println(currentPlayer.getName() + "获胜!");
break;
}
}
}
// 获取下一个出牌玩家
public static Player getNextPlayer(Player player1, Player player2, Player player3, Player landlord) {
if (landlord == player1) {
return player2.getCards().size() > player3.getCards().size() ? player2 : player3;
} else if (landlord == player2) {
return player1.getCards().size() > player3.getCards().size() ? player1 : player3;
} else {
return player1.getCards().size() > player2.getCards().size() ? player1 : player2;
}
}
// 计算得分
public static void calculateScore(Player player1, Player player2, Player player3, Player landlord) {
int score = landlord.getScore();
if (player1 == landlord) {
player2.setScore(player2.getScore() - score);
player3.setScore(player3.getScore() - score);
} else if (player2 == landlord) {
player1.setScore(player1.getScore() - score);
player3.setScore(player3.getScore() - score);
} else {
player1.setScore(player1.getScore() - score);
player2.setScore(player2.getScore() - score);
}
System.out.println("游戏结束,得分情况:");
System.out.println(player1.getName() + ":" + player1.getScore() + "分");
System.out.println(player2.getName() + ":" + player2.getScore() + "分");
System.out.println(player3.getName() + ":" + player3.getScore() + "分");
}
}
class Player {
private String name;
private List<String> cards;
private int score;
public Player(String name) {
this.name = name;
this.cards = new ArrayList<>();
this.score = 0;
}
public void addCard(String card) {
this.cards.add(card);
}
public void removeCards(List<String> playCards) {
this.cards.removeAll(playCards);
}
public boolean checkCards(List<String> playCards) {
// TODO: 实现出牌规则判断
return true;
}
public String getName() {
return name;
}
public List<String> getCards() {
return cards;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
```
上面的代码实现了斗地主游戏的基本流程,包括初始化牌堆、发牌、叫地主、出牌、计算得分等功能。需要注意的是,其中`checkCards`方法需要实现出牌规则的判断,这里只是留出了一个TODO注释。如果您需要实现更完整的斗地主游戏,还需要考虑以下方面:
- 实现出牌规则的判断
- 实现AI玩家的出牌行为
- 实现更完善的UI界面
- 实现游戏回放等高级功能
希望对您有所帮助!
相关推荐
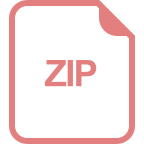














