答辩问使用spring boot和,vue和mysql如何实现商品分类
时间: 2023-07-14 22:13:11 浏览: 174
使用 Spring Boot 和 MySQL 可以通过 JPA 或 MyBatis 实现持久层的操作,使用 Vue 框架实现前端页面的渲染和交互。
针对商品分类,可以使用如下的实现方式:
1. 创建一个 Category 实体类,包含分类的 ID、名称、父级 ID 等属性;
2. 设计 CategoryRepository 接口并实现,用于实现对 Category 实体的 CRUD 操作;
3. 在后端控制器中,通过 CategoryRepository 实现对分类信息的增删改查等操作;
4. 在前端页面中,使用 Vue 调用后端接口,实现对分类信息的展示和操作。
具体实现步骤如下:
1. 创建 Category 实体类,包含以下属性:
```java
@Entity
@Table(name = "category")
public class Category {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id; // 分类 ID
private String name; // 分类名称
@Column(name = "parent_id")
private Long parentId; // 父级分类 ID
// 省略 getter 和 setter 方法
}
```
2. 设计 CategoryRepository 接口并实现,用于实现对 Category 实体的 CRUD 操作,例如:
```java
@Repository
public interface CategoryRepository extends JpaRepository<Category, Long> {
List<Category> findByParentId(Long parentId);
List<Category> findByParentIdIsNull();
}
```
3. 在后端控制器中,通过 CategoryRepository 实现对分类信息的增删改查等操作,例如:
```java
@RestController
@RequestMapping("/api/categories")
public class CategoryController {
@Autowired
private CategoryRepository categoryRepository;
@GetMapping("")
public List<Category> getAllCategories() {
return categoryRepository.findAll();
}
@GetMapping("/{id}")
public Category getCategoryById(@PathVariable Long id) {
return categoryRepository.findById(id).orElse(null);
}
@PostMapping("")
public Category createCategory(@RequestBody Category category) {
return categoryRepository.save(category);
}
@PutMapping("/{id}")
public Category updateCategory(@PathVariable Long id, @RequestBody Category category) {
Category savedCategory = categoryRepository.findById(id).orElse(null);
if (savedCategory != null) {
savedCategory.setName(category.getName());
savedCategory.setParentId(category.getParentId());
return categoryRepository.save(savedCategory);
}
return null;
}
@DeleteMapping("/{id}")
public void deleteCategory(@PathVariable Long id) {
categoryRepository.deleteById(id);
}
@GetMapping("/root")
public List<Category> getRootCategories() {
return categoryRepository.findByParentIdIsNull();
}
@GetMapping("/{id}/children")
public List<Category> getCategoryChildren(@PathVariable Long id) {
return categoryRepository.findByParentId(id);
}
}
```
4. 在前端页面中,使用 Vue 调用后端接口,实现对分类信息的展示和操作,例如:
```html
<template>
<div>
<h2>分类列表</h2>
<ul>
<li v-for="(category, index) in categories" :key="index">
{{ category.name }}
<button @click="editCategory(category)">编辑</button>
<button @click="deleteCategory(category)">删除</button>
<button v-if="category.children" @click="toggleChildren(category)">
{{ category.showChildren ? '隐藏子分类' : '显示子分类' }}
</button>
<ul v-if="category.children && category.showChildren">
<li v-for="(child, index) in category.children" :key="index">
{{ child.name }}
<button @click="editCategory(child)">编辑</button>
<button @click="deleteCategory(child)">删除</button>
</li>
</ul>
</li>
</ul>
<h2>添加分类</h2>
<form @submit.prevent="addCategory">
<label>名称:</label>
<input type="text" v-model="newCategoryName">
<label>父级分类 ID:</label>
<input type="text" v-model="newCategoryParentId" placeholder="可不填">
<button type="submit">添加</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
categories: [],
newCategoryName: '',
newCategoryParentId: null,
};
},
methods: {
getCategories() {
axios.get('/api/categories').then(response => {
this.categories = response.data;
});
},
addCategory() {
let category = {
name: this.newCategoryName,
parentId: this.newCategoryParentId,
};
axios.post('/api/categories', category).then(response => {
this.getCategories();
this.newCategoryName = '';
this.newCategoryParentId = null;
});
},
editCategory(category) {
// TODO: 编辑分类信息
},
deleteCategory(category) {
axios.delete(`/api/categories/${category.id}`).then(() => {
this.getCategories();
});
},
toggleChildren(category) {
if (!category.children) {
axios.get(`/api/categories/${category.id}/children`).then(response => {
category.children = response.data;
category.showChildren = true;
});
} else {
category.showChildren = !category.showChildren;
}
},
},
mounted() {
this.getCategories();
},
};
</script>
```
阅读全文
相关推荐
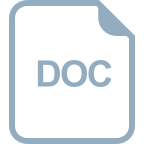
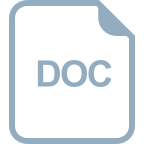
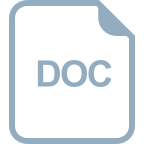




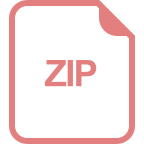
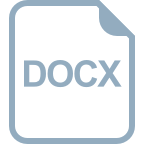
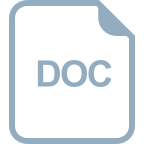
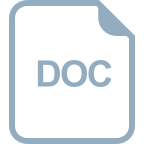

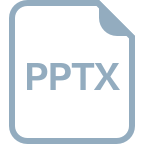
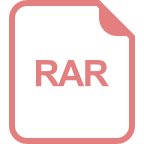
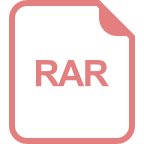
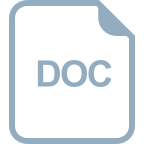
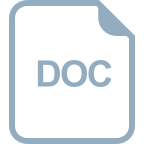
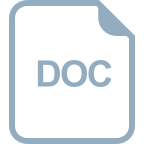