有一个一维数组用于存放10个学生的成绩,要求编写三个函数分别求学生的平均成绩,求10个学生的最高分,求10个学生的最低分,在主函数中调用这三个函数得到结果。
时间: 2024-05-12 17:18:17 浏览: 29
好的,我明白了。以下是针对您的问题的三个函数的实现:
```python
def average_score(scores):
total = sum(scores)
return total / len(scores)
def max_score(scores):
return max(scores)
def min_score(scores):
return min(scores)
```
这些函数中,average_score() 函数用于计算学生的平均分,max_score() 函数用于计算学生的最高分,min_score() 函数用于计算学生的最低分。
现在,在主函数中,可以这样来调用这些函数:
```python
scores = [80, 90, 70, 60, 85, 93, 77, 88, 75, 82]
avg = average_score(scores)
max = max_score(scores)
min = min_score(scores)
print("平均分:", avg)
print("最高分:", max)
print("最低分:", min)
```
这里,我们假设这 10 名学生的成绩已经存在一个列表(scores)中。
当然,您可以使用不同的成绩来测试这些函数。
相关问题
有一个一维数组用于存放10个学生的成绩,要求编写三个函数分别求学生的平均成绩,求10个学生的最高分,求10个学生的最低分,在主函数中调用这三个函数得到结果要求C语言编写
好的,我可以回答这个问题。以下是三个函数的代码:
// 求平均成绩
float average(float scores[]) {
float sum = ;
for (int i = ; i < 10; i++) {
sum += scores[i];
}
return sum / 10;
}
// 求最高分
float max(float scores[]) {
float max_score = scores[];
for (int i = 1; i < 10; i++) {
if (scores[i] > max_score) {
max_score = scores[i];
}
}
return max_score;
}
// 求最低分
float min(float scores[]) {
float min_score = scores[];
for (int i = 1; i < 10; i++) {
if (scores[i] < min_score) {
min_score = scores[i];
}
}
return min_score;
}
在主函数中调用这三个函数,可以这样写:
int main() {
float scores[10] = {80, 90, 70, 85, 95, 75, 60, 88, 92, 78};
float avg = average(scores);
float max_score = max(scores);
float min_score = min(scores);
printf("平均成绩为:%.2f\n", avg);
printf("最高分为:%.2f\n", max_score);
printf("最低分为:%.2f\n", min_score);
return ;
}
这样就可以得到学生的平均成绩、最高分和最低分了。
c语言有一个一维数组用于存放10个学生的成绩,要求编写三个函数分别求学生的平均成绩,求10个学生的最高分,求10个学生的最低分,在主函数中调用这三个函数得到结果
好的,这是一个关于 C 语言的问题。对于这个问题,我可以给出以下三个函数的实现:
1. 求学生的平均成绩:
```c
float average(float scores[]) {
float sum = ;
for(int i = ; i < 10; i++) {
sum += scores[i];
}
return sum / 10;
}
```
2. 求10个学生的最高分:
```c
float max(float scores[]) {
float max_score = scores[];
for(int i = 1; i < 10; i++) {
if(scores[i] > max_score) {
max_score = scores[i];
}
}
return max_score;
}
```
3. 求10个学生的最低分:
```c
float min(float scores[]) {
float min_score = scores[];
for(int i = 1; i < 10; i++) {
if(scores[i] < min_score) {
min_score = scores[i];
}
}
return min_score;
}
```
在主函数中,可以这样调用这三个函数:
```c
int main() {
float scores[10] = {90, 80, 70, 60, 50, 40, 30, 20, 10, };
float avg = average(scores);
float max_score = max(scores);
float min_score = min(scores);
printf("平均成绩:%f\n", avg);
printf("最高分:%f\n", max_score);
printf("最低分:%f\n", min_score);
return ;
}
```
这样就可以得到学生的平均成绩、最高分和最低分了。
阅读全文
相关推荐
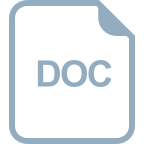
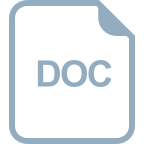
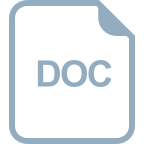







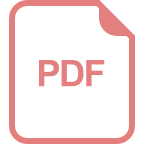



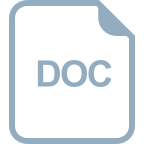
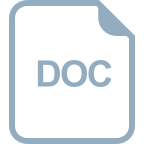
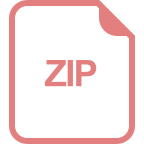