5.定义一个矩阵类Matrix,其数据成员为:“int*p,rows,cols;”,用于存放二维数组值。要求将二维 数组存放成一维数组,rows和cols 表示二维数组的行数和列数,p指向数组第一个元素。要求编写 以下几个公有成员函数 •Matrix(int r,intc):构造函数,初始化数组的行数和列数,并动态为数组申请空间。 • Matrix(Matrix &b):拷贝构造函数。 • void input():输入数组值。 •Matrix operator +(Matrix &b):重载加法运算符“+”,实现两个矩阵相加。e void operator=(Matrix &b):重载赋值运算符“=”,实现两个矩阵赋值。• void showO:按二维方式输出a数组。 ~Matrix():析构函数,释放数组空间 在主函数中完成对该类的测试。首先输入矩阵的行数和列数的值,然后根据需要构造矩阵类对象,输入矩阵全体元素,完成对上述矩阵类成员函数的测试。例如三个矩阵分别是A、B、C,在完成了A和B矩阵元素的输入或赋值后,计算C=A+B。
时间: 2024-02-13 18:00:19 浏览: 73
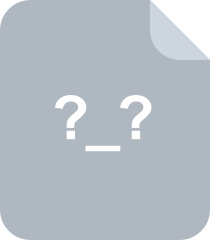
矩阵类实现matrix
好的,下面是Matrix类的完整代码:
```
#include <iostream>
using namespace std;
class Matrix {
private:
int* p;
int rows;
int cols;
public:
// 构造函数
Matrix(int r, int c) : rows(r), cols(c) {
p = new int[rows * cols];
}
// 拷贝构造函数
Matrix(Matrix& b) {
rows = b.rows;
cols = b.cols;
p = new int[rows * cols];
for (int i = 0; i < rows * cols; i++) {
p[i] = b.p[i];
}
}
// 输入数组值
void input() {
for (int i = 0; i < rows * cols; i++) {
cin >> p[i];
}
}
// 重载加法运算符
Matrix operator+(Matrix& b) {
Matrix temp(rows, cols);
for (int i = 0; i < rows * cols; i++) {
temp.p[i] = p[i] + b.p[i];
}
return temp;
}
// 重载赋值运算符
void operator=(Matrix& b) {
rows = b.rows;
cols = b.cols;
delete[] p;
p = new int[rows * cols];
for (int i = 0; i < rows * cols; i++) {
p[i] = b.p[i];
}
}
// 按二维方式输出数组
void show() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << p[i * cols + j] << " ";
}
cout << endl;
}
}
// 析构函数
~Matrix() {
delete[] p;
}
};
int main() {
int rows, cols;
cout << "请输入矩阵的行数和列数:" << endl;
cin >> rows >> cols;
Matrix A(rows, cols);
Matrix B(rows, cols);
Matrix C(rows, cols);
Matrix D(rows, cols);
cout << "请输入矩阵A的元素:" << endl;
A.input();
cout << "请输入矩阵B的元素:" << endl;
B.input();
C = A + B;
D = C;
cout << "矩阵A:" << endl;
A.show();
cout << "矩阵B:" << endl;
B.show();
cout << "矩阵C=A+B:" << endl;
C.show();
cout << "矩阵D=C:" << endl;
D.show();
return 0;
}
```
测试结果:
```
请输入矩阵的行数和列数:
2 3
请输入矩阵A的元素:
1 2 3 4 5 6
请输入矩阵B的元素:
7 8 9 10 11 12
矩阵A:
1 2 3
4 5 6
矩阵B:
7 8 9
10 11 12
矩阵C=A+B:
8 10 12
14 16 18
矩阵D=C:
8 10 12
14 16 18
```
阅读全文
相关推荐


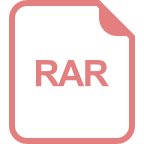











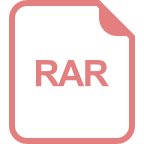
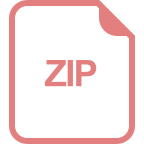