4. 建立一个字符串类 String, 要求如下: 1)私有数据成员: • char *str:字符串的首地址。 •int ten:宇符串的长度值。 2)公有成员函数: •构造两数:动态申请存储字符串所需的内存空间,并且既能用参数指定的字符串也能用默认值 NULL 进行宇符串的初始化。 • 拷贝构造两数。 •析构两数:释放字符串所使用的动态内存空问。 • void set(char *s):为字符串赋新值。 •void shovQ:显示字符串。 •int getlen0:返回字符串的长度。 • void delchar(char ch):删除字符串中出现的所有ch字符。 • String operator -(char ch):重载“一”运算符,实现删除字符串中出现的所有c字符(如str-h)。 • String & operator =(String &):重载“=”运算符,实现宇符串的直接赋值。 • String & operator +=(String &):重载“+=”运算符,实现两宇符串的拼接。 •bool operator =-(String &):重载 运算符,进行两宇符串的相等性比较,相等返回 true, 杏则返回 false。 3)友元函数: • friend String operator +(String &, String &):重载“+〞运算符,实现两字符串的相加 请编写完整的程序,测试类的各成员两数的正确性。 ’5.定义一个矩阵类 Matrix,其数据成员为: "int.*p, rows, cols;" ,用于存放二维数组值。要水将 数组存放成一维数组,rows-和cols 表示二维数组的行数和列数,p指向数组第一个元素。要求编写 以下几个公有成员兩数。 ••Matrix(int r, int c):构造两数,初始化如组的行数和列数,并动态为数组中请空间
时间: 2024-01-21 18:17:35 浏览: 163
1. String 类的实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
int len;
public:
String() {
len = 0;
str = new char[1];
str[0] = '\0';
}
String(const char *s) {
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
String(const String &s) {
len = s.len;
str = new char[len + 1];
strcpy(str, s.str);
}
~String() {
delete[] str;
}
void set(char *s) {
delete[] str;
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
void show() {
cout << str << endl;
}
int getlen() {
return len;
}
void delchar(char ch) {
int index = 0;
for (int i = 0; i < len; i++) {
if (str[i] != ch) {
str[index++] = str[i];
}
}
str[index] = '\0';
len = index;
}
String operator-(char ch) {
String temp(*this);
temp.delchar(ch);
return temp;
}
String& operator=(String &s) {
if (this == &s) {
return *this;
}
delete[] str;
len = s.len;
str = new char[len + 1];
strcpy(str, s.str);
return *this;
}
String& operator+=(String &s) {
len += s.len;
char *temp = new char[len + 1];
strcpy(temp, str);
strcat(temp, s.str);
delete[] str;
str = temp;
return *this;
}
bool operator==(String &s) {
if (len != s.len) {
return false;
}
return strcmp(str, s.str) == 0;
}
friend String operator+(String &s1, String &s2) {
String temp;
temp.len = s1.len + s2.len;
temp.str = new char[temp.len + 1];
strcpy(temp.str, s1.str);
strcat(temp.str, s2.str);
return temp;
}
};
int main() {
String s1("hello");
String s2;
s2 = s1;
s2 -= 'l';
s1 += s2;
s1.show();
if (s1 == s2) {
cout << "s1 equals s2" << endl;
} else {
cout << "s1 does not equal s2" << endl;
}
String s3 = s1 + s2;
s3.show();
return 0;
}
```
2. Matrix 类的实现:
```cpp
#include <iostream>
using namespace std;
class Matrix {
private:
int *p;
int rows, cols;
public:
Matrix(int r, int c) {
rows = r;
cols = c;
p = new int[rows * cols];
}
~Matrix() {
delete[] p;
}
int& operator()(int i, int j) {
return *(p + i * cols + j);
}
Matrix operator+(Matrix &m) {
Matrix temp(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
temp(i, j) = (*this)(i, j) + m(i, j);
}
}
return temp;
}
void show() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << (*this)(i, j) << " ";
}
cout << endl;
}
}
};
int main() {
Matrix m1(3, 3), m2(3, 3);
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
m1(i, j) = i + j;
m2(i, j) = i - j;
}
}
Matrix m3 = m1 + m2;
m3.show();
return 0;
}
```
阅读全文
相关推荐
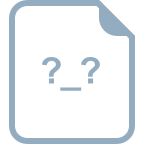












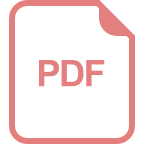
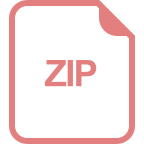